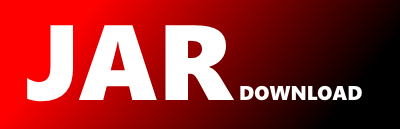
com.github.javaclub.mq.common.netty.NettyClient Maven / Gradle / Ivy
package com.github.javaclub.mq.common.netty;
import java.util.concurrent.TimeUnit;
import io.netty.bootstrap.Bootstrap;
import io.netty.channel.Channel;
import io.netty.channel.ChannelFuture;
import io.netty.channel.ChannelInitializer;
import io.netty.channel.ChannelOption;
import io.netty.channel.EventLoopGroup;
import io.netty.channel.nio.NioEventLoopGroup;
import io.netty.channel.socket.SocketChannel;
import io.netty.channel.socket.nio.NioSocketChannel;
public class NettyClient {
public static Bootstrap createBootstrap(final ChannelPipelineFactory channelPipelineFactory) {
Bootstrap b = new Bootstrap();
EventLoopGroup workerGroup = new NioEventLoopGroup();
b.group(workerGroup);
b.channel(NioSocketChannel.class);
b.option(ChannelOption.SO_KEEPALIVE, true);
b.option(ChannelOption.CONNECT_TIMEOUT_MILLIS, 3000);
b.option(ChannelOption.TCP_NODELAY, true);
b.handler(new ChannelInitializer() {
@Override
public void initChannel(SocketChannel ch) throws Exception {
if (channelPipelineFactory != null) {
channelPipelineFactory.initChannelPipeline(ch.pipeline());
}
}
});
return b;
}
public static Channel get(Bootstrap bootstrap, String host, int port) throws InterruptedException {
ChannelFuture f = bootstrap.connect(host, port);
if (!f.awaitUninterruptibly(3, TimeUnit.SECONDS)) {
f.cancel(true);
return null;
}
return f.channel();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy