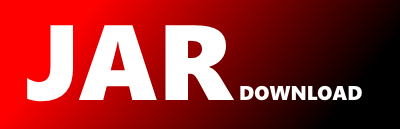
com.github.javaclub.mq.common.netty.NettyServer Maven / Gradle / Ivy
package com.github.javaclub.mq.common.netty;
import io.netty.bootstrap.ServerBootstrap;
import io.netty.channel.ChannelInitializer;
import io.netty.channel.ChannelOption;
import io.netty.channel.EventLoopGroup;
import io.netty.channel.nio.NioEventLoopGroup;
import io.netty.channel.socket.SocketChannel;
import io.netty.channel.socket.nio.NioServerSocketChannel;
public class NettyServer {
private int port;
private ChannelPipelineFactory channelPipelineFactory;
public NettyServer(int port, ChannelPipelineFactory channelPipelineFactory) {
this.port = port;
this.channelPipelineFactory = channelPipelineFactory;
}
public void start() throws InterruptedException {
EventLoopGroup bossGroup = new NioEventLoopGroup();
EventLoopGroup workerGroup = new NioEventLoopGroup();
ServerBootstrap b = new ServerBootstrap();
b.group(bossGroup, workerGroup).channel(NioServerSocketChannel.class).childHandler(new ChannelInitializer() {
@Override
public void initChannel(SocketChannel ch) throws Exception {
if (channelPipelineFactory != null) {
channelPipelineFactory.initChannelPipeline(ch.pipeline());
}
}
}).option(ChannelOption.SO_BACKLOG, 128)
.childOption(ChannelOption.SO_KEEPALIVE, true)
.childOption(ChannelOption.TCP_NODELAY, true);
// Bind and start to accept incoming connections.
b.bind(port).sync();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy