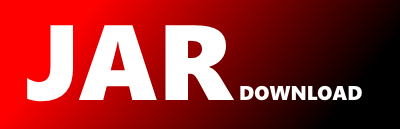
com.github.javaclub.mq.common.netty.protocol.CheckMsgProtocol Maven / Gradle / Ivy
package com.github.javaclub.mq.common.netty.protocol;
import io.netty.buffer.ByteBuf;
/**
* broker向producer发送check包,check本地事务是否成功
*/
public class CheckMsgProtocol extends AbstractProtocol {
private static byte code = CodeProtocol.check_msg_transaction;
private int length; // header + body
private int headerLength;
private byte[] header;
private byte[] body;
public void encode(ByteBuf out) {
super.setCode(code);
super.encode(out);
out.writeInt(length);
out.writeInt(headerLength);
out.writeBytes(header);
out.writeBytes(body);
}
public int decode(ByteBuf in) {
int readindex = super.decode(in);
if (readindex != -1) {
if (in.readableBytes() >= 8) {
readindex = in.readerIndex();
length = in.readInt();
headerLength = in.readInt();
if (in.readableBytes() >= length) {
byte[] header = new byte[headerLength];
in.readBytes(header);
this.header = header;
byte[] body = new byte[length - headerLength];
in.readBytes(body);
this.body = body;
return 0;
} else {
in.readerIndex(readindex); // 恢复readindex
return -1;
}
} else {
in.readerIndex(readindex); // 恢复readindex
return -1;
}
}
return -1;
}
public byte getCode() {
return code;
}
public void setCode(byte code) {
CheckMsgProtocol.code = code;
}
public int getLength() {
return length;
}
public void setLength(int length) {
this.length = length;
}
public int getHeaderLength() {
return headerLength;
}
public void setHeaderLength(int headerLength) {
this.headerLength = headerLength;
}
public byte[] getHeader() {
return header;
}
public void setHeader(byte[] header) {
this.header = header;
}
public byte[] getBody() {
return body;
}
public void setBody(byte[] body) {
this.body = body;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy