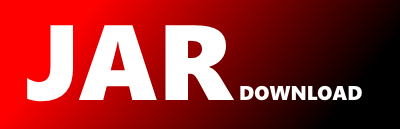
spring.auto.boot.processor.SsoAppProcessor Maven / Gradle / Ivy
/*
* @(#)SsoAppProcessor.java 2021-10-27
*
* Copyright (c) 2021. All Rights Reserved.
*
*/
package spring.auto.boot.processor;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.BeansException;
import org.springframework.beans.factory.config.BeanDefinition;
import org.springframework.beans.factory.config.ConfigurableListableBeanFactory;
import org.springframework.beans.factory.support.BeanDefinitionRegistry;
import org.springframework.beans.factory.support.BeanDefinitionRegistryPostProcessor;
import org.springframework.beans.factory.support.RootBeanDefinition;
import org.springframework.web.servlet.mvc.method.annotation.RequestMappingHandlerMapping;
import com.github.javaclub.sso.SSOConstants;
import com.github.javaclub.sso.SSOConstants.Client;
import com.github.javaclub.sso.common.util.Utils;
import com.github.javaclub.sso.exception.SSOException;
import com.github.javaclub.toolbox.conf.CompositeAppConfigProperties;
import spring.auto.boot.config.ClientAppWebConfig;
import spring.auto.boot.controller.SsoClientController;
import spring.auto.boot.controller.SsoServerApiController;
/**
* SsoAppProcessor
*
* @author Gerald Chen
* @version $Id: SsoAppProcessor.java 2021-10-27 10:52:49 Exp $
*/
public class SsoAppProcessor implements BeanDefinitionRegistryPostProcessor {
private static final Logger log = LoggerFactory.getLogger(SsoAppProcessor.class);
public void postProcessBeanFactory(ConfigurableListableBeanFactory beanFactory) throws BeansException {
}
public void postProcessBeanDefinitionRegistry(BeanDefinitionRegistry registry) throws BeansException {
if (!isInWebMvc()) {
throw new SSOException("必须在web容器内(包括定时器、dubbo应用)");
}
if (isSsoServerApp()) {
BeanDefinition controllerBeanDefinition = new RootBeanDefinition(SsoServerApiController.class);
registry.registerBeanDefinition(SsoServerApiController.class.getSimpleName().toLowerCase(), controllerBeanDefinition);
log.info("SsoServer SsoServerApiController init start.");
return;
}
BeanDefinition clientAppConfigBean = new RootBeanDefinition(ClientAppWebConfig.class);
registry.registerBeanDefinition(ClientAppWebConfig.class.getSimpleName().toLowerCase(), clientAppConfigBean);
log.info("SsoClient ClientAppWebConfig init start.");
BeanDefinition controllerBeanDefinition = new RootBeanDefinition(SsoClientController.class);
registry.registerBeanDefinition(SsoClientController.class.getSimpleName().toLowerCase(), controllerBeanDefinition);
log.info("SsoClient SsoClientController init start.");
}
boolean isInWebMvc() {
try {
Class.forName(RequestMappingHandlerMapping.class.getName());
return true;
} catch (Throwable e) {
return false;
}
}
boolean isSsoServerApp() {
String appRole = CompositeAppConfigProperties.getInstance().getValue(Client.ROLE);
return Utils.equalsIgnoreCase(SSOConstants.SSO, appRole);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy