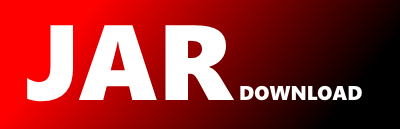
com.github.javaclub.sword.util.AppProperties Maven / Gradle / Ivy
package com.github.javaclub.sword.util;
import java.util.Collection;
import java.util.HashMap;
import java.util.Map;
import java.util.Properties;
import java.util.Set;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.BeansException;
import org.springframework.beans.factory.config.ConfigurableListableBeanFactory;
import org.springframework.beans.factory.config.PropertyPlaceholderConfigurer;
/**
* AppProperties 应用属性配置文件装载
*
* @author Gerald Chen
* @version $Id: AppProperties.java 2017年9月20日 22:49:24 Exp $
*/
public class AppProperties extends PropertyPlaceholderConfigurer implements Map {
private static final Logger logger = LoggerFactory.getLogger(AppProperties.class);
private static Map ctxPropertiesMap;
@Override
protected void processProperties(ConfigurableListableBeanFactory beanFactoryToProcess, Properties props)
throws BeansException {
super.processProperties(beanFactoryToProcess, props);
if (ctxPropertiesMap != null) {
logger.warn("The property map will be override!");
}
ctxPropertiesMap = new HashMap();
for (Object key : props.keySet()) {
String keyStr = key.toString();
String value = props.getProperty(keyStr);
ctxPropertiesMap.put(keyStr, value);
}
}
public static String getString(String name) {
if (ctxPropertiesMap == null) {
ctxPropertiesMap = new HashMap();
}
return (String) ctxPropertiesMap.get(name);
}
public static boolean getBoolean(String name, boolean defaultValue) {
String v = getString(name);
if (v == null) {
return defaultValue;
}
try {
return Boolean.parseBoolean(v);
} catch (Exception e) {
}
return defaultValue;
}
public static int getIntValue(String name, int defaultValue) {
String v = getString(name);
if (v == null) {
return defaultValue;
}
try {
return Integer.parseInt(v);
} catch (Exception e) {
}
return defaultValue;
}
public static long getLongValue(String name, long defaultValue) {
String v = getString(name);
if (v == null) {
return defaultValue;
}
try {
return Long.parseLong(v);
} catch (Exception e) {
}
return defaultValue;
}
public static short getShortValue(String name, short defaultValue) {
String v = getString(name);
if (v == null) {
return defaultValue;
}
try {
return Short.parseShort(v);
} catch (Exception e) {
}
return defaultValue;
}
public static double getDoubleValue(String name, double defaultValue) {
String v = getString(name);
if (v == null) {
return defaultValue;
}
try {
return Double.parseDouble(v);
} catch (Exception e) {
}
return defaultValue;
}
public static float getFloatValue(String name, float defaultValue) {
String v = getString(name);
if (v == null) {
return defaultValue;
}
try {
return Float.parseFloat(v);
} catch (Exception e) {
}
return defaultValue;
}
public int size() {
return ctxPropertiesMap.size();
}
public boolean isEmpty() {
return ctxPropertiesMap.isEmpty();
}
public boolean containsKey(Object key) {
return ctxPropertiesMap.containsKey(key);
}
public Set keySet() {
return ctxPropertiesMap.keySet();
}
public String get(Object key) {
return ctxPropertiesMap.get(key);
}
public Collection values() {
return ctxPropertiesMap.values();
}
public Set> entrySet() {
return ctxPropertiesMap.entrySet();
}
public boolean containsValue(Object value) {
return null == value ? false : ctxPropertiesMap.containsValue(value.toString());
}
public String put(String key, String value) {
throw new UnsupportedOperationException();
}
public String remove(Object key) {
throw new UnsupportedOperationException();
}
public void putAll(Map extends String, ? extends String> m) {
throw new UnsupportedOperationException();
}
public void clear() {
throw new UnsupportedOperationException();
}
// env
static final String ENV_DAILY = "daily";
static final String ENV_GRAY = "gray";
static final String ENV_ONLINE = "online";
/**
* 获取当前环境
*/
public static String getEnv() {
return null == AppProperties.getString("env") ? AppProperties.getString("config.env") : AppProperties.getString("env");
}
/**
* 日常环境
*/
public static boolean isDaily() {
return ENV_DAILY.equals(getEnv());
}
/**
* 灰度环境
*/
public static boolean isGray() {
return ENV_GRAY.equals(getEnv());
}
/**
* 线上环境
*/
public static boolean isOnline() {
return ENV_ONLINE.equals(getEnv());
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy