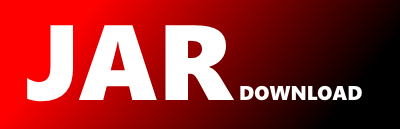
com.github.javaclub.sword.App Maven / Gradle / Ivy
The newest version!
package com.github.javaclub.sword;
import java.io.BufferedReader;
import java.io.ByteArrayInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.StringReader;
import java.util.ArrayList;
import java.util.List;
import java.util.Properties;
import org.springframework.util.FileCopyUtils;
import com.github.javaclub.sword.core.Numbers;
import com.github.javaclub.sword.core.Strings;
import com.github.javaclub.sword.util.FileUtil;
/**
* App
*
* @author Gerald Chen
* @version $Id: App.java 2017年5月15日 19:00:11 Exp $
*/
public final class App {
static final String groupId = "com.github.javaclub";
static final String artifactId= "sword";
public static Thread currentThread() {
return Thread.currentThread();
}
public static void sleep(long min, long max) {
try {
Thread.sleep(Numbers.random(min, max));
} catch (InterruptedException e) {
// ignore;
}
}
public static void sleep(long mills) {
try {
Thread.sleep(mills);
} catch (InterruptedException e) {
// ignore;
}
}
public static void sleep(long baseMills, long intervalPerTimes, int tryTimes) {
long min = baseMills;
try {
min = baseMills + intervalPerTimes*tryTimes;
min = min > (Long.MAX_VALUE - 100000L) ? (Long.MAX_VALUE - 100000L) : min;
} catch (Exception ex) {
min = Long.MAX_VALUE - 200000L;
}
long max = min + 60*1000L;
try {
Thread.sleep(Numbers.random(min, max));
} catch (InterruptedException e) {
// ignore;
}
}
public static String getCurrentJarVersion() {
Properties pom = null;
try {
pom = getJarProperties(groupId, artifactId);
} catch (IOException e) {
}
return null == pom ? null : pom.getProperty("version");
}
public static Properties getJarProperties(String groupId, String artifactId) throws IOException {
Properties packageProperties = new Properties();
String mainfestFile = "META-INF/MANIFEST.MF";
String mavenPomFile = Strings.format("META-INF/maven/{}/{}/pom.properties", groupId, artifactId);
InputStream pomResource = FileUtil.getClasspathStream(mavenPomFile);
if (null != pomResource) {
// #Generated by Maven
// #Wed Jul 22 13:09:50 CST 2015
// version=1.0.5-SNAPSHOT
// groupId=com.github.javaclub
// artifactId=sword
byte[] pomData = FileCopyUtils.copyToByteArray(pomResource);
packageProperties.load(new ByteArrayInputStream(pomData));
BufferedReader br = new BufferedReader(new StringReader(new String(pomData, "utf-8")));
List lines = new ArrayList();
for (String line = br.readLine(); line != null; line = br.readLine()) {
lines.add(line);
}
// try to get package time
if (lines.size() >= 2 && lines.get(1).startsWith("#")) {
String time = lines.get(1).substring(1);
packageProperties.put("packageTime", time);
}
}
// Manifest-Version: 1.0
// Archiver-Version: Plexus Archiver
// Built-By: hongyuanabc
// Created-By: Apache Maven 3.3.1
// Build-Jdk: 1.8.0_45
InputStream mainfestResource = FileUtil.getClasspathStream(mainfestFile);
if (null != mainfestResource) {
byte[] mainfestData = FileCopyUtils.copyToByteArray(mainfestResource);
BufferedReader br = new BufferedReader(new StringReader(new String(mainfestData)));
for (String line = br.readLine(); line != null; line = br.readLine()) {
String[] split = Strings.split(line, ':');
if (split != null && split.length == 2) {
packageProperties.put(split[0].trim(), split[1].trim());
}
}
}
return packageProperties;
}
public static void main( String[] args ) throws IOException {
System.out.println(getCurrentJarVersion());
System.out.println(getJarProperties("com.github.javaclub", "configcenter-client"));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy