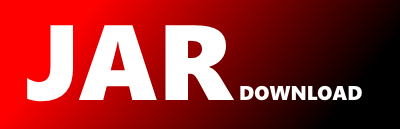
com.github.javaclub.toolbox.crypt.SimpleEncryptor Maven / Gradle / Ivy
package com.github.javaclub.toolbox.crypt;
import com.github.javaclub.toolbox.ToolBox.Strings;
public class SimpleEncryptor {
private SimpleEncryptor() {}
public final static boolean isTooSimple(String password) {
if(Strings.isBlank(password) || 6 > password.trim().length()) {
return true;
}
String pwd = password.trim();
if(6 == pwd.length() && Strings.isNumeric(pwd)) {
return true;
}
return false;
}
/**
* Encrypts the specified string.
*
* @param input the string to encrypt.
* @return the encrypted string.
*/
public static String encrypt(String input) {
StringBuilder sResult = new StringBuilder();
for (int i = 0; i < input.length(); i++) {
int iAsc = input.charAt(i);
if (iAsc >= 97 && iAsc <= 109) {
iAsc = iAsc + 13; //a ... m inclusive become n ... z
}
else if (iAsc >= 110 && iAsc <= 122) {
iAsc = iAsc - 13; //n ... z inclusive become a ... m
}
else if (iAsc >= 65 && iAsc <= 77) {
iAsc = iAsc + 13; //A ... M inclusive become N ... Z
}
else if (iAsc >= 78 && iAsc <= 90) {
iAsc = iAsc - 13; //N ... Z inclusive become A ... M
}
sResult.append((char) iAsc);
}
return sResult.toString();
}
/**
* Decrypts the encrypted string.
*
* @param encrypted a encrypted string.
* @return the original string before encrypted.
*/
public static String decrypt(String encrypted) {
return encrypt(encrypted);
}
public static void main(String[] args) {
String s = "haha我";
String encrypt = SimpleEncryptor.encrypt(s);
System.out.println(encrypt);
String decrypt = SimpleEncryptor.decrypt(encrypt);
System.out.println(decrypt);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy