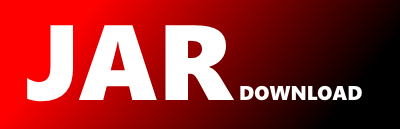
g0001_0100.s0051_n_queens.Solution.dart Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of leetcode-in-all Show documentation
Show all versions of leetcode-in-all Show documentation
104 LeetCode algorithm problem solutions
The newest version!
// #Hard #Top_100_Liked_Questions #Array #Backtracking #Big_O_Time_O(N!)_Space_O(N)
// #2024_10_04_Time_328_ms_(86.67%)_Space_149.1_MB_(73.33%)
class Solution {
List> solveNQueens(int n) {
final List> board = List.generate(n, (_) => List.filled(n, '.'));
final List> ans = [];
backtrack(board, ans, 0, 0, n);
return ans;
}
bool isSafe(List> board, int row, int col) {
for (int i = 0; i < row; i++) {
if (board[i][col] == 'Q') {
return false;
}
}
for (int i = row, j = col; i >= 0 && j >= 0; i--, j--) {
if (board[i][j] == 'Q') {
return false;
}
}
for (int i = row, j = col; i >= 0 && j < board.length; i--, j++) {
if (board[i][j] == 'Q') {
return false;
}
}
return true;
}
void backtrack(List> board, List> ans, int row, int col, int queens) {
if (row == queens) {
ans.add(convert(board));
return;
}
for (int col = 0; col < board.length; col++) {
if (isSafe(board, row, col)) {
board[row][col] = 'Q';
backtrack(board, ans, row + 1, col, queens);
board[row][col] = '.';
}
}
}
List convert(List> board) {
final List ans = [];
for (int i = 0; i < board.length; i++) {
final row = board[i].join('');
ans.add(row);
}
return ans;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy