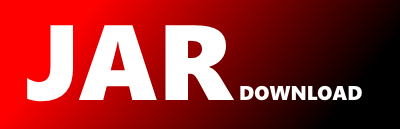
g0001_0100.s0051_n_queens.Solution Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of leetcode-in-java Show documentation
Show all versions of leetcode-in-java Show documentation
Java-based LeetCode algorithm problem solutions, regularly updated
The newest version!
package g0001_0100.s0051_n_queens;
// #Hard #Top_100_Liked_Questions #Array #Backtracking #Big_O_Time_O(N!)_Space_O(N)
// #2024_11_11_Time_1_ms_(99.77%)_Space_44.8_MB_(61.16%)
import java.util.ArrayList;
import java.util.List;
public class Solution {
public List> solveNQueens(int n) {
char[][] board = new char[n][n];
for (int i = 0; i < n; i++) {
for (int j = 0; j < n; j++) {
board[i][j] = '.';
}
}
List> res = new ArrayList<>();
int[] leftRow = new int[n];
int[] upperDiagonal = new int[2 * n - 1];
int[] lowerDiagonal = new int[2 * n - 1];
solve(0, board, res, leftRow, lowerDiagonal, upperDiagonal);
return res;
}
void solve(
int col,
char[][] board,
List> res,
int[] leftRow,
int[] lowerDiagonal,
int[] upperDiagonal) {
if (col == board.length) {
res.add(construct(board));
return;
}
for (int row = 0; row < board.length; row++) {
if (leftRow[row] == 0
&& lowerDiagonal[row + col] == 0
&& upperDiagonal[board.length - 1 + col - row] == 0) {
board[row][col] = 'Q';
leftRow[row] = 1;
lowerDiagonal[row + col] = 1;
upperDiagonal[board.length - 1 + col - row] = 1;
solve(col + 1, board, res, leftRow, lowerDiagonal, upperDiagonal);
board[row][col] = '.';
leftRow[row] = 0;
lowerDiagonal[row + col] = 0;
upperDiagonal[board.length - 1 + col - row] = 0;
}
}
}
List construct(char[][] board) {
List res = new ArrayList<>();
for (char[] chars : board) {
String s = new String(chars);
res.add(s);
}
return res;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy