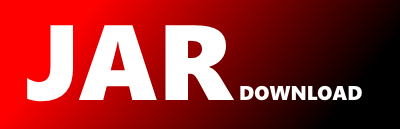
g0001_0100.s0048_rotate_image.Solution Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of leetcode-in-java21 Show documentation
Show all versions of leetcode-in-java21 Show documentation
Java-based LeetCode algorithm problem solutions, regularly updated
package g0001_0100.s0048_rotate_image;
// #Medium #Top_100_Liked_Questions #Top_Interview_Questions #Array #Math #Matrix
// #Data_Structure_II_Day_3_Array #Programming_Skills_II_Day_7 #Udemy_2D_Arrays/Matrix
// #Big_O_Time_O(n^2)_Space_O(1) #2023_08_11_Time_0_ms_(100.00%)_Space_41.5_MB_(34.96%)
/**
* 48 - Rotate Image\.
*
* Medium
*
* You are given an `n x n` 2D `matrix` representing an image, rotate the image by **90** degrees (clockwise).
*
* You have to rotate the image [**in-place** ](https://en.wikipedia.org/wiki/In-place_algorithm), which means you have to modify the input 2D matrix directly. **DO NOT** allocate another 2D matrix and do the rotation.
*
* **Example 1:**
*
* 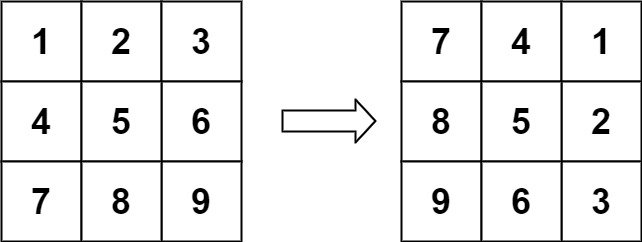
*
* **Input:** matrix = \[\[1,2,3],[4,5,6],[7,8,9]]
*
* **Output:** [[7,4,1],[8,5,2],[9,6,3]]
*
* **Example 2:**
*
* 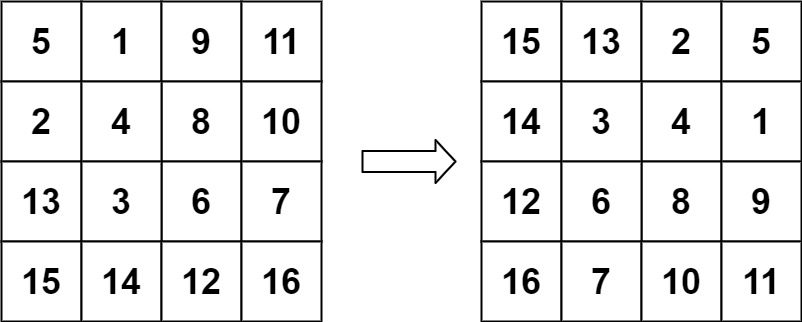
*
* **Input:** matrix = \[\[5,1,9,11],[2,4,8,10],[13,3,6,7],[15,14,12,16]]
*
* **Output:** [[15,13,2,5],[14,3,4,1],[12,6,8,9],[16,7,10,11]]
*
* **Example 3:**
*
* **Input:** matrix = \[\[1]]
*
* **Output:** [[1]]
*
* **Example 4:**
*
* **Input:** matrix = \[\[1,2],[3,4]]
*
* **Output:** [[3,1],[4,2]]
*
* **Constraints:**
*
* * `matrix.length == n`
* * `matrix[i].length == n`
* * `1 <= n <= 20`
* * `-1000 <= matrix[i][j] <= 1000`
**/
public class Solution {
public void rotate(int[][] matrix) {
int n = matrix.length;
for (int i = 0; i < n / 2; i++) {
for (int j = i; j < n - i - 1; j++) {
int[][] pos =
new int[][] {
{i, j}, {j, n - 1 - i}, {n - 1 - i, n - 1 - j}, {n - 1 - j, i}
};
int t = matrix[pos[0][0]][pos[0][1]];
for (int k = 1; k < pos.length; k++) {
int temp = matrix[pos[k][0]][pos[k][1]];
matrix[pos[k][0]][pos[k][1]] = t;
t = temp;
}
matrix[pos[0][0]][pos[0][1]] = t;
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy