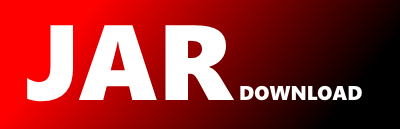
g0001_0100.s0050_powx_n.Solution Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of leetcode-in-java21 Show documentation
Show all versions of leetcode-in-java21 Show documentation
Java-based LeetCode algorithm problem solutions, regularly updated
package g0001_0100.s0050_powx_n;
// #Medium #Top_Interview_Questions #Math #Recursion #Udemy_Integers
// #2023_08_11_Time_0_ms_(100.00%)_Space_41.2_MB_(14.99%)
/**
* 50 - Pow(x, n)\.
*
* Medium
*
* Implement [pow(x, n)](http://www.cplusplus.com/reference/valarray/pow/), which calculates `x` raised to the power `n` (i.e., xn
).
*
* **Example 1:**
*
* **Input:** x = 2.00000, n = 10
*
* **Output:** 1024.00000
*
* **Example 2:**
*
* **Input:** x = 2.10000, n = 3
*
* **Output:** 9.26100
*
* **Example 3:**
*
* **Input:** x = 2.00000, n = -2
*
* **Output:** 0.25000
*
* **Explanation:** 2\-2 = 1/22 = 1/4 = 0.25
*
* **Constraints:**
*
* * `-100.0 < x < 100.0`
* * -231 <= n <= 231-1
* * -104 <= xn <= 104
**/
public class Solution {
public double myPow(double x, int n) {
long nn = n;
double res = 1;
if (n < 0) {
nn *= -1;
}
while (nn > 0) {
if (nn % 2 == 1) {
nn--;
res *= x;
} else {
x *= x;
nn /= 2;
}
}
if (n < 0) {
return 1.0 / res;
}
return res;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy