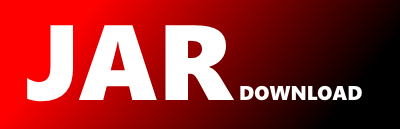
g0001_0100.s0057_insert_interval.Solution Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of leetcode-in-java21 Show documentation
Show all versions of leetcode-in-java21 Show documentation
Java-based LeetCode algorithm problem solutions, regularly updated
package g0001_0100.s0057_insert_interval;
// #Medium #Array #Level_2_Day_17_Interval #2023_08_11_Time_0_ms_(100.00%)_Space_43.7_MB_(95.60%)
import java.util.Arrays;
/**
* 57 - Insert Interval\.
*
* Medium
*
* You are given an array of non-overlapping intervals `intervals` where intervals[i] = [starti, endi]
represent the start and the end of the ith
interval and `intervals` is sorted in ascending order by starti
. You are also given an interval `newInterval = [start, end]` that represents the start and end of another interval.
*
* Insert `newInterval` into `intervals` such that `intervals` is still sorted in ascending order by starti
and `intervals` still does not have any overlapping intervals (merge overlapping intervals if necessary).
*
* Return `intervals` _after the insertion_.
*
* **Example 1:**
*
* **Input:** intervals = \[\[1,3],[6,9]], newInterval = [2,5]
*
* **Output:** [[1,5],[6,9]]
*
* **Example 2:**
*
* **Input:** intervals = \[\[1,2],[3,5],[6,7],[8,10],[12,16]], newInterval = [4,8]
*
* **Output:** [[1,2],[3,10],[12,16]]
*
* **Explanation:** Because the new interval `[4,8]` overlaps with `[3,5],[6,7],[8,10]`.
*
* **Example 3:**
*
* **Input:** intervals = [], newInterval = [5,7]
*
* **Output:** [[5,7]]
*
* **Example 4:**
*
* **Input:** intervals = \[\[1,5]], newInterval = [2,3]
*
* **Output:** [[1,5]]
*
* **Example 5:**
*
* **Input:** intervals = \[\[1,5]], newInterval = [2,7]
*
* **Output:** [[1,7]]
*
* **Constraints:**
*
* * 0 <= intervals.length <= 104
* * `intervals[i].length == 2`
* * 0 <= starti <= endi <= 105
* * `intervals` is sorted by starti
in **ascending** order.
* * `newInterval.length == 2`
* * 0 <= start <= end <= 105
**/
public class Solution {
public int[][] insert(int[][] intervals, int[] newInterval) {
int n = intervals.length;
int l = 0;
int r = n - 1;
while (l < n && newInterval[0] > intervals[l][1]) {
l++;
}
while (r >= 0 && newInterval[1] < intervals[r][0]) {
r--;
}
int[][] res = new int[l + n - r][2];
for (int i = 0; i < l; i++) {
res[i] = Arrays.copyOf(intervals[i], intervals[i].length);
}
res[l][0] = Math.min(newInterval[0], l == n ? newInterval[0] : intervals[l][0]);
res[l][1] = Math.max(newInterval[1], r == -1 ? newInterval[1] : intervals[r][1]);
for (int i = l + 1, j = r + 1; j < n; i++, j++) {
res[i] = intervals[j];
}
return res;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy