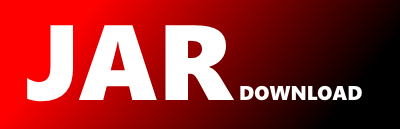
g0001_0100.s0062_unique_paths.Solution Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of leetcode-in-java21 Show documentation
Show all versions of leetcode-in-java21 Show documentation
Java-based LeetCode algorithm problem solutions, regularly updated
package g0001_0100.s0062_unique_paths;
// #Medium #Top_100_Liked_Questions #Top_Interview_Questions #Dynamic_Programming #Math
// #Combinatorics #Algorithm_II_Day_13_Dynamic_Programming #Dynamic_Programming_I_Day_15
// #Level_1_Day_11_Dynamic_Programming #Big_O_Time_O(m*n)_Space_O(m*n)
// #2023_08_11_Time_0_ms_(100.00%)_Space_39.2_MB_(67.74%)
/**
* 62 - Unique Paths\.
*
* Medium
*
* A robot is located at the top-left corner of a `m x n` grid (marked 'Start' in the diagram below).
*
* The robot can only move either down or right at any point in time. The robot is trying to reach the bottom-right corner of the grid (marked 'Finish' in the diagram below).
*
* How many possible unique paths are there?
*
* **Example 1:**
*
* 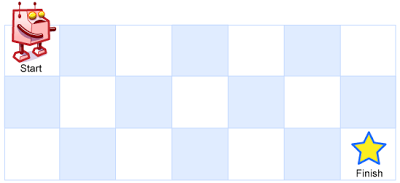
*
* **Input:** m = 3, n = 7
*
* **Output:** 28
*
* **Example 2:**
*
* **Input:** m = 3, n = 2
*
* **Output:** 3
*
* **Explanation:**
*
* From the top-left corner, there are a total of 3 ways to reach the bottom-right corner:
* 1. Right -> Down -> Down
* 2. Down -> Down -> Right
* 3. Down -> Right -> Down
*
* **Example 3:**
*
* **Input:** m = 7, n = 3
*
* **Output:** 28
*
* **Example 4:**
*
* **Input:** m = 3, n = 3
*
* **Output:** 6
*
* **Constraints:**
*
* * `1 <= m, n <= 100`
* * It's guaranteed that the answer will be less than or equal to 2 * 109
.
**/
public class Solution {
public int uniquePaths(int m, int n) {
int[][] dp = new int[m][n];
for (int i = 0; i < m; i++) {
dp[i][0] = 1;
}
for (int j = 0; j < n; j++) {
dp[0][j] = 1;
}
for (int i = 1; i < m; i++) {
for (int j = 1; j < n; j++) {
dp[i][j] = dp[i - 1][j] + dp[i][j - 1];
}
}
return dp[m - 1][n - 1];
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy