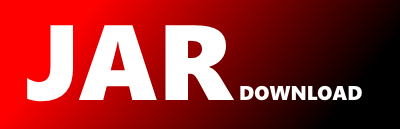
g0001_0100.s0063_unique_paths_ii.Solution Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of leetcode-in-java21 Show documentation
Show all versions of leetcode-in-java21 Show documentation
Java-based LeetCode algorithm problem solutions, regularly updated
package g0001_0100.s0063_unique_paths_ii;
// #Medium #Array #Dynamic_Programming #Matrix #Dynamic_Programming_I_Day_15
// #2023_08_11_Time_0_ms_(100.00%)_Space_40.6_MB_(73.18%)
/**
* 63 - Unique Paths II\.
*
* Medium
*
* A robot is located at the top-left corner of a `m x n` grid (marked 'Start' in the diagram below).
*
* The robot can only move either down or right at any point in time. The robot is trying to reach the bottom-right corner of the grid (marked 'Finish' in the diagram below).
*
* Now consider if some obstacles are added to the grids. How many unique paths would there be?
*
* An obstacle and space is marked as `1` and `0` respectively in the grid.
*
* **Example 1:**
*
* 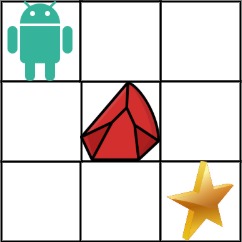
*
* **Input:** obstacleGrid = \[\[0,0,0],[0,1,0],[0,0,0]]
*
* **Output:** 2
*
* **Explanation:** There is one obstacle in the middle of the 3x3 grid above. There are two ways to reach the bottom-right corner: 1. Right -> Right -> Down -> Down 2. Down -> Down -> Right -> Right
*
* **Example 2:**
*
* 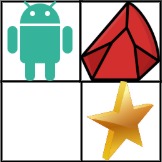
*
* **Input:** obstacleGrid = \[\[0,1],[0,0]]
*
* **Output:** 1
*
* **Constraints:**
*
* * `m == obstacleGrid.length`
* * `n == obstacleGrid[i].length`
* * `1 <= m, n <= 100`
* * `obstacleGrid[i][j]` is `0` or `1`.
**/
public class Solution {
public int uniquePathsWithObstacles(int[][] obstacleGrid) {
// if start point has obstacle, there's no path
if (obstacleGrid[0][0] == 1) {
return 0;
}
obstacleGrid[0][0] = 1;
int m = obstacleGrid.length;
int n = obstacleGrid[0].length;
for (int i = 1; i < m; i++) {
if (obstacleGrid[i][0] == 1) {
obstacleGrid[i][0] = 0;
} else {
obstacleGrid[i][0] = obstacleGrid[i - 1][0];
}
}
for (int j = 1; j < n; j++) {
if (obstacleGrid[0][j] == 1) {
obstacleGrid[0][j] = 0;
} else {
obstacleGrid[0][j] = obstacleGrid[0][j - 1];
}
}
for (int i = 1; i < m; i++) {
for (int j = 1; j < n; j++) {
if (obstacleGrid[i][j] == 1) {
obstacleGrid[i][j] = 0;
} else {
obstacleGrid[i][j] = obstacleGrid[i - 1][j] + obstacleGrid[i][j - 1];
}
}
}
return obstacleGrid[m - 1][n - 1];
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy