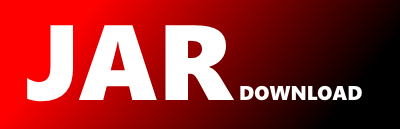
g0001_0100.s0071_simplify_path.Solution Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of leetcode-in-java21 Show documentation
Show all versions of leetcode-in-java21 Show documentation
Java-based LeetCode algorithm problem solutions, regularly updated
package g0001_0100.s0071_simplify_path;
// #Medium #String #Stack #2023_08_11_Time_2_ms_(99.80%)_Space_41.7_MB_(99.37%)
import java.util.ArrayDeque;
import java.util.Deque;
/**
* 71 - Simplify Path\.
*
* Medium
*
* Given a string `path`, which is an **absolute path** (starting with a slash `'/'`) to a file or directory in a Unix-style file system, convert it to the simplified **canonical path**.
*
* In a Unix-style file system, a period `'.'` refers to the current directory, a double period `'..'` refers to the directory up a level, and any multiple consecutive slashes (i.e. `'//'`) are treated as a single slash `'/'`. For this problem, any other format of periods such as `'...'` are treated as file/directory names.
*
* The **canonical path** should have the following format:
*
* * The path starts with a single slash `'/'`.
* * Any two directories are separated by a single slash `'/'`.
* * The path does not end with a trailing `'/'`.
* * The path only contains the directories on the path from the root directory to the target file or directory (i.e., no period `'.'` or double period `'..'`)
*
* Return _the simplified **canonical path**_.
*
* **Example 1:**
*
* **Input:** path = "/home/"
*
* **Output:** "/home"
*
* **Explanation:** Note that there is no trailing slash after the last directory name.
*
* **Example 2:**
*
* **Input:** path = "/../"
*
* **Output:** "/"
*
* **Explanation:** Going one level up from the root directory is a no-op, as the root level is the highest level you can go.
*
* **Example 3:**
*
* **Input:** path = "/home//foo/"
*
* **Output:** "/home/foo"
*
* **Explanation:** In the canonical path, multiple consecutive slashes are replaced by a single one.
*
* **Example 4:**
*
* **Input:** path = "/a/./b/../../c/"
*
* **Output:** "/c"
*
* **Constraints:**
*
* * `1 <= path.length <= 3000`
* * `path` consists of English letters, digits, period `'.'`, slash `'/'` or `'_'`.
* * `path` is a valid absolute Unix path.
**/
public class Solution {
public String simplifyPath(String path) {
Deque stk = new ArrayDeque<>();
int start = 0;
while (start < path.length()) {
while (start < path.length() && path.charAt(start) == '/') {
start++;
}
int end = start;
while (end < path.length() && path.charAt(end) != '/') {
end++;
}
String s = path.substring(start, end);
if (s.equals("..")) {
if (!stk.isEmpty()) {
stk.pop();
}
} else if (!s.equals(".") && !s.equals("")) {
stk.push(s);
}
start = end + 1;
}
StringBuilder ans = new StringBuilder();
while (!stk.isEmpty()) {
ans.insert(0, stk.pop());
ans.insert(0, "/");
}
return ans.length() > 0 ? ans.toString() : "/";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy