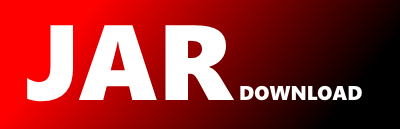
g0101_0200.s0133_clone_graph.Solution Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of leetcode-in-java21 Show documentation
Show all versions of leetcode-in-java21 Show documentation
Java-based LeetCode algorithm problem solutions, regularly updated
package g0101_0200.s0133_clone_graph;
// #Medium #Hash_Table #Depth_First_Search #Breadth_First_Search #Graph #Udemy_Graph
// #2022_06_24_Time_45_ms_(29.80%)_Space_42.7_MB_(77.96%)
import com_github_leetcode.Node;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.Map;
/**
* 133 - Clone Graph\.
*
* Medium
*
* Given a reference of a node in a **[connected](https://en.wikipedia.org/wiki/Connectivity_(graph_theory)#Connected_graph)** undirected graph.
*
* Return a [**deep copy** ](https://en.wikipedia.org/wiki/Object_copying#Deep_copy) (clone) of the graph.
*
* Each node in the graph contains a value (`int`) and a list (`List[Node]`) of its neighbors.
*
* class Node { public int val; public List<Node> neighbors; }
*
* **Test case format:**
*
* For simplicity, each node's value is the same as the node's index (1-indexed). For example, the first node with `val == 1`, the second node with `val == 2`, and so on. The graph is represented in the test case using an adjacency list.
*
* **An adjacency list** is a collection of unordered **lists** used to represent a finite graph. Each list describes the set of neighbors of a node in the graph.
*
* The given node will always be the first node with `val = 1`. You must return the **copy of the given node** as a reference to the cloned graph.
*
* **Example 1:**
*
* 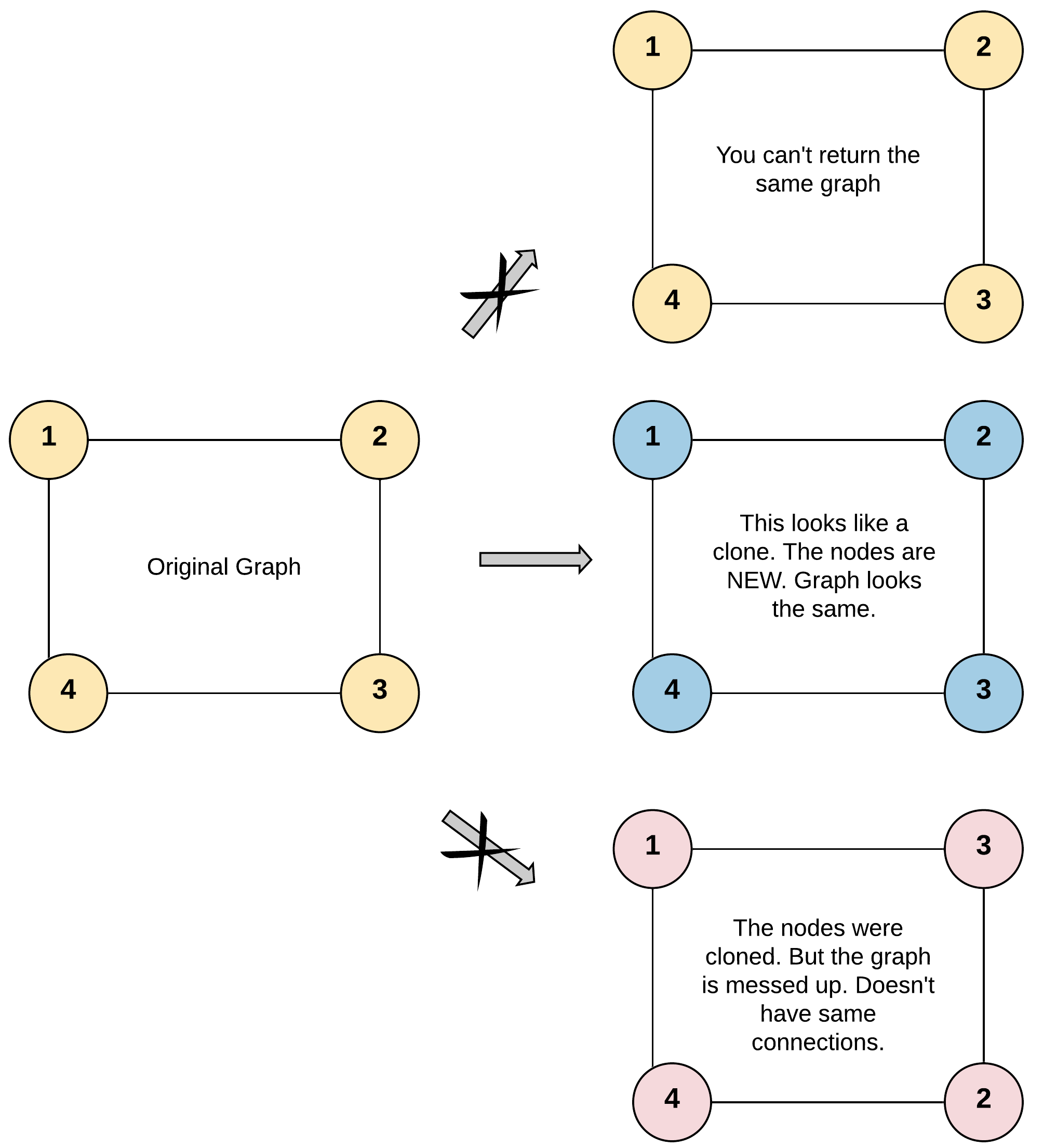
*
* **Input:** adjList = \[\[2,4],[1,3],[2,4],[1,3]]
*
* **Output:** [[2,4],[1,3],[2,4],[1,3]]
*
* **Explanation:**
*
* There are 4 nodes in the graph.
* 1st node (val = 1)'s neighbors are 2nd node (val = 2) and 4th node (val = 4).
* 2nd node (val = 2)'s neighbors are 1st node (val = 1) and 3rd node (val = 3).
* 3rd node (val = 3)'s neighbors are 2nd node (val = 2) and 4th node (val = 4).
* 4th node (val = 4)'s neighbors are 1st node (val = 1) and 3rd node (val = 3).
*
* **Example 2:**
*
* 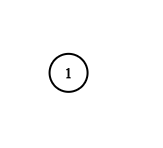
*
* **Input:** adjList = \[\[]]
*
* **Output:** [ []]
*
* **Explanation:** Note that the input contains one empty list. The graph consists of only one node with val = 1 and it does not have any neighbors.
*
* **Example 3:**
*
* **Input:** adjList = []
*
* **Output:** []
*
* **Explanation:** This an empty graph, it does not have any nodes.
*
* **Example 4:**
*
* 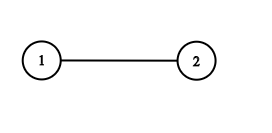
*
* **Input:** adjList = \[\[2],[1]]
*
* **Output:** [[2],[1]]
*
* **Constraints:**
*
* * The number of nodes in the graph is in the range `[0, 100]`.
* * `1 <= Node.val <= 100`
* * `Node.val` is unique for each node.
* * There are no repeated edges and no self-loops in the graph.
* * The Graph is connected and all nodes can be visited starting from the given node.
**/
public class Solution {
public Node cloneGraph(Node node) {
return cloneGraph(node, new HashMap<>());
}
private Node cloneGraph(Node node, Map processedNodes) {
if (node == null) {
return null;
} else if (processedNodes.get(node) != null) {
return processedNodes.get(node);
}
Node newNode = new Node();
processedNodes.put(node, newNode);
newNode.val = node.val;
newNode.neighbors = new ArrayList<>();
for (Node neighbor : node.neighbors) {
Node clonedNeighbor = cloneGraph(neighbor, processedNodes);
if (clonedNeighbor != null) {
newNode.neighbors.add(clonedNeighbor);
}
}
return newNode;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy