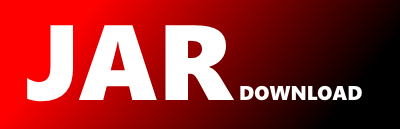
g0101_0200.s0141_linked_list_cycle.Solution Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of leetcode-in-java21 Show documentation
Show all versions of leetcode-in-java21 Show documentation
Java-based LeetCode algorithm problem solutions, regularly updated
package g0101_0200.s0141_linked_list_cycle;
// #Easy #Top_100_Liked_Questions #Top_Interview_Questions #Hash_Table #Two_Pointers #Linked_List
// #Data_Structure_I_Day_7_Linked_List #Udemy_Linked_List #Big_O_Time_O(N)_Space_O(1)
// #2022_06_24_Time_0_ms_(100.00%)_Space_45.5_MB_(68.52%)
import com_github_leetcode.ListNode;
/*
* Definition for singly-linked list.
* class ListNode {
* int val;
* ListNode next;
* ListNode(int x) {
* val = x;
* next = null;
* }
* }
*/
/**
* 141 - Linked List Cycle\.
*
* Easy
*
* Given `head`, the head of a linked list, determine if the linked list has a cycle in it.
*
* There is a cycle in a linked list if there is some node in the list that can be reached again by continuously following the `next` pointer. Internally, `pos` is used to denote the index of the node that tail's `next` pointer is connected to. **Note that `pos` is not passed as a parameter**.
*
* Return `true` _if there is a cycle in the linked list_. Otherwise, return `false`.
*
* **Example 1:**
*
* 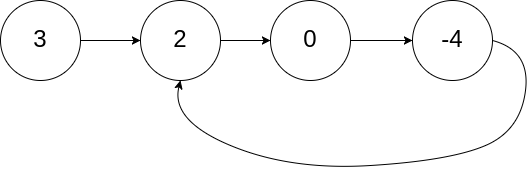
*
* **Input:** head = [3,2,0,-4], pos = 1
*
* **Output:** true
*
* **Explanation:** There is a cycle in the linked list, where the tail connects to the 1st node (0-indexed).
*
* **Example 2:**
*
* 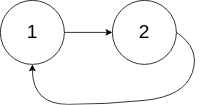
*
* **Input:** head = [1,2], pos = 0
*
* **Output:** true
*
* **Explanation:** There is a cycle in the linked list, where the tail connects to the 0th node.
*
* **Example 3:**
*
* 
*
* **Input:** head = [1], pos = -1
*
* **Output:** false
*
* **Explanation:** There is no cycle in the linked list.
*
* **Constraints:**
*
* * The number of the nodes in the list is in the range [0, 104]
.
* * -105 <= Node.val <= 105
* * `pos` is `-1` or a **valid index** in the linked-list.
*
* **Follow up:** Can you solve it using `O(1)` (i.e. constant) memory?
**/
public class Solution {
public boolean hasCycle(ListNode head) {
if (head == null) {
return false;
}
ListNode fast = head.next;
ListNode slow = head;
while (fast != null && fast.next != null) {
if (fast == slow) {
return true;
}
fast = fast.next.next;
slow = slow.next;
}
return false;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy