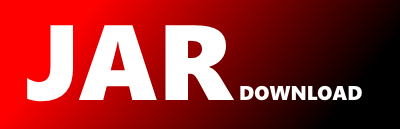
g0101_0200.s0189_rotate_array.Solution Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of leetcode-in-java21 Show documentation
Show all versions of leetcode-in-java21 Show documentation
Java-based LeetCode algorithm problem solutions, regularly updated
package g0101_0200.s0189_rotate_array;
// #Medium #Top_100_Liked_Questions #Top_Interview_Questions #Array #Math #Two_Pointers
// #Algorithm_I_Day_2_Two_Pointers #Udemy_Arrays #Big_O_Time_O(n)_Space_O(1)
// #2022_06_27_Time_0_ms_(100.00%)_Space_58_MB_(96.22%)
/**
* 189 - Rotate Array\.
*
* Medium
*
* Given an array, rotate the array to the right by `k` steps, where `k` is non-negative.
*
* **Example 1:**
*
* **Input:** nums = [1,2,3,4,5,6,7], k = 3
*
* **Output:** [5,6,7,1,2,3,4]
*
* **Explanation:**
*
* rotate 1 steps to the right: [7,1,2,3,4,5,6]
* rotate 2 steps to the right: [6,7,1,2,3,4,5]
* rotate 3 steps to the right: [5,6,7,1,2,3,4]
*
* **Example 2:**
*
* **Input:** nums = [-1,-100,3,99], k = 2
*
* **Output:** [3,99,-1,-100]
*
* **Explanation:**
*
* rotate 1 steps to the right: [99,-1,-100,3]
* rotate 2 steps to the right: [3,99,-1,-100]
*
* **Constraints:**
*
* * 1 <= nums.length <= 105
* * -231 <= nums[i] <= 231 - 1
* * 0 <= k <= 105
*
* **Follow up:**
*
* * Try to come up with as many solutions as you can. There are at least **three** different ways to solve this problem.
* * Could you do it in-place with `O(1)` extra space?
**/
public class Solution {
private void reverse(int[] nums, int l, int r) {
while (l <= r) {
int temp = nums[l];
nums[l] = nums[r];
nums[r] = temp;
l++;
r--;
}
}
public void rotate(int[] nums, int k) {
int n = nums.length;
int t = n - (k % n);
reverse(nums, 0, t - 1);
reverse(nums, t, n - 1);
reverse(nums, 0, n - 1);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy