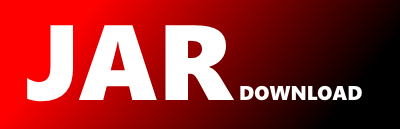
g0201_0300.s0202_happy_number.Solution Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of leetcode-in-java21 Show documentation
Show all versions of leetcode-in-java21 Show documentation
Java-based LeetCode algorithm problem solutions, regularly updated
package g0201_0300.s0202_happy_number;
// #Easy #Top_Interview_Questions #Hash_Table #Math #Two_Pointers #Algorithm_II_Day_21_Others
// #Programming_Skills_I_Day_4_Loop #Level_2_Day_1_Implementation/Simulation
// #2022_06_28_Time_1_ms_(98.59%)_Space_41_MB_(64.25%)
/**
* 202 - Happy Number\.
*
* Easy
*
* Write an algorithm to determine if a number `n` is happy.
*
* A **happy number** is a number defined by the following process:
*
* * Starting with any positive integer, replace the number by the sum of the squares of its digits.
* * Repeat the process until the number equals 1 (where it will stay), or it **loops endlessly in a cycle** which does not include 1.
* * Those numbers for which this process **ends in 1** are happy.
*
* Return `true` _if_ `n` _is a happy number, and_ `false` _if not_.
*
* **Example 1:**
*
* **Input:** n = 19
*
* **Output:** true
*
* **Explanation:**
*
* 12 + 92 = 82
*
* 82 + 22 = 68
*
* 62 + 82 = 100
*
* 12 + 02 + 02 = 1
*
* **Example 2:**
*
* **Input:** n = 2
*
* **Output:** false
*
* **Constraints:**
*
* * 1 <= n <= 231 - 1
**/
public class Solution {
public boolean isHappy(int n) {
boolean happy;
int a = n;
int rem;
int sum = 0;
if (a == 1 || a == 7) {
happy = true;
} else if (a > 1 && a < 10) {
happy = false;
} else {
while (a != 0) {
rem = a % 10;
sum = sum + (rem * rem);
a = a / 10;
}
if (sum != 1) {
happy = isHappy(sum);
} else {
happy = true;
}
}
return happy;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy