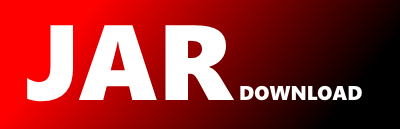
g0201_0300.s0216_combination_sum_iii.Solution Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of leetcode-in-java21 Show documentation
Show all versions of leetcode-in-java21 Show documentation
Java-based LeetCode algorithm problem solutions, regularly updated
package g0201_0300.s0216_combination_sum_iii;
// #Medium #Array #Backtracking #Udemy_Backtracking/Recursion
// #2022_07_02_Time_1_ms_(81.35%)_Space_41.8_MB_(46.36%)
import java.util.ArrayList;
import java.util.List;
/**
* 216 - Combination Sum III\.
*
* Medium
*
* Find all valid combinations of `k` numbers that sum up to `n` such that the following conditions are true:
*
* * Only numbers `1` through `9` are used.
* * Each number is used **at most once**.
*
* Return _a list of all possible valid combinations_. The list must not contain the same combination twice, and the combinations may be returned in any order.
*
* **Example 1:**
*
* **Input:** k = 3, n = 7
*
* **Output:** [[1,2,4]]
*
* **Explanation:**
*
* 1 + 2 + 4 = 7
* There are no other valid combinations.
*
* **Example 2:**
*
* **Input:** k = 3, n = 9
*
* **Output:** [[1,2,6],[1,3,5],[2,3,4]]
*
* **Explanation:**
*
* 1 + 2 + 6 = 9
* 1 + 3 + 5 = 9
* 2 + 3 + 4 = 9
* There are no other valid combinations.
*
* **Example 3:**
*
* **Input:** k = 4, n = 1
*
* **Output:** []
*
* **Explanation:**
*
* There are no valid combinations.
* Using 4 different numbers in the range [1,9], the smallest sum we can get is 1+2+3+4 = 10 and since 10 > 1, there are no valid combination.
*
* **Example 4:**
*
* **Input:** k = 3, n = 2
*
* **Output:** []
*
* **Explanation:** There are no valid combinations.
*
* **Example 5:**
*
* **Input:** k = 9, n = 45
*
* **Output:** [[1,2,3,4,5,6,7,8,9]]
*
* **Explanation:**
*
* 1 + 2 + 3 + 4 + 5 + 6 + 7 + 8 + 9 = 45
* There are no other valid combinations.
*
* **Constraints:**
*
* * `2 <= k <= 9`
* * `1 <= n <= 60`
**/
@SuppressWarnings("java:S5413")
public class Solution {
public List> combinationSum3(int k, int n) {
List> res = new ArrayList<>();
solve(k, n, new ArrayList<>(), res, 0, 1);
return res;
}
private void solve(
int k, int target, List temp, List> res, int sum, int start) {
if (sum == target && temp.size() == k) {
res.add(new ArrayList<>(temp));
return;
}
if (temp.size() >= k) {
return;
}
if (sum > target) {
return;
}
for (int i = start; i <= 9; i++) {
temp.add(i);
solve(k, target, temp, res, sum + i, i + 1);
temp.remove(temp.size() - 1);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy