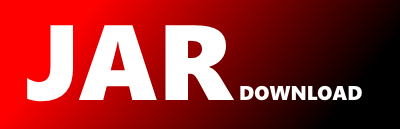
g0301_0400.s0330_patching_array.Solution Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of leetcode-in-java21 Show documentation
Show all versions of leetcode-in-java21 Show documentation
Java-based LeetCode algorithm problem solutions, regularly updated
package g0301_0400.s0330_patching_array;
// #Hard #Array #Greedy #2022_07_09_Time_1_ms_(60.00%)_Space_44.3_MB_(27.06%)
/**
* 330 - Patching Array\.
*
* Hard
*
* Given a sorted integer array `nums` and an integer `n`, add/patch elements to the array such that any number in the range `[1, n]` inclusive can be formed by the sum of some elements in the array.
*
* Return _the minimum number of patches required_.
*
* **Example 1:**
*
* **Input:** nums = [1,3], n = 6
*
* **Output:** 1
*
* **Explanation:**
*
* Combinations of nums are [1], [3], [1,3], which form possible sums of: 1, 3, 4.
* Now if we add/patch 2 to nums, the combinations are: [1], [2], [3], [1,3], [2,3], [1,2,3].
* Possible sums are 1, 2, 3, 4, 5, 6, which now covers the range [1, 6].
* So we only need 1 patch.
*
* **Example 2:**
*
* **Input:** nums = [1,5,10], n = 20
*
* **Output:** 2
*
* **Explanation:** The two patches can be [2, 4].
*
* **Example 3:**
*
* **Input:** nums = [1,2,2], n = 5
*
* **Output:** 0
*
* **Constraints:**
*
* * `1 <= nums.length <= 1000`
* * 1 <= nums[i] <= 104
* * `nums` is sorted in **ascending order**.
* * 1 <= n <= 231 - 1
**/
public class Solution {
public int minPatches(int[] nums, int n) {
int res = 0;
long sum = 0;
int i = 0;
while (sum < n) {
// required number
long req = sum + 1;
if (i < nums.length && nums[i] <= req) {
sum += nums[i++];
} else {
sum += req;
res++;
}
}
return res;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy