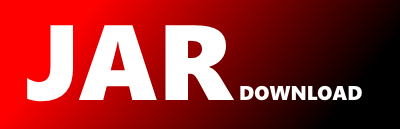
g0301_0400.s0338_counting_bits.Solution Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of leetcode-in-java21 Show documentation
Show all versions of leetcode-in-java21 Show documentation
Java-based LeetCode algorithm problem solutions, regularly updated
package g0301_0400.s0338_counting_bits;
// #Easy #Top_100_Liked_Questions #Dynamic_Programming #Bit_Manipulation #Udemy_Bit_Manipulation
// #Big_O_Time_O(num)_Space_O(num) #2022_07_10_Time_2_ms_(86.73%)_Space_48.3_MB_(31.59%)
/**
* 338 - Counting Bits\.
*
* Easy
*
* Given an integer `n`, return _an array_ `ans` _of length_ `n + 1` _such that for each_ `i` (`0 <= i <= n`)_,_ `ans[i]` _is the **number of**_ `1`_**'s** in the binary representation of_ `i`.
*
* **Example 1:**
*
* **Input:** n = 2
*
* **Output:** [0,1,1]
*
* **Explanation:**
*
* 0 --> 0
* 1 --> 1
* 2 --> 10
*
* **Example 2:**
*
* **Input:** n = 5
*
* **Output:** [0,1,1,2,1,2]
*
* **Explanation:**
*
* 0 --> 0
* 1 --> 1
* 2 --> 10
* 3 --> 11
* 4 --> 100
* 5 --> 101
*
* **Constraints:**
*
* * 0 <= n <= 105
*
* **Follow up:**
*
* * It is very easy to come up with a solution with a runtime of `O(n log n)`. Can you do it in linear time `O(n)` and possibly in a single pass?
* * Can you do it without using any built-in function (i.e., like `__builtin_popcount` in C++)?
**/
public class Solution {
public int[] countBits(int num) {
int[] result = new int[num + 1];
int borderPos = 1;
int incrPos = 1;
for (int i = 1; i < result.length; i++) {
// when we reach pow of 2 , reset borderPos and incrPos
if (incrPos == borderPos) {
result[i] = 1;
incrPos = 1;
borderPos = i;
} else {
result[i] = 1 + result[incrPos++];
}
}
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy