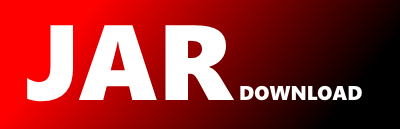
g0401_0500.s0409_longest_palindrome.Solution Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of leetcode-in-java21 Show documentation
Show all versions of leetcode-in-java21 Show documentation
Java-based LeetCode algorithm problem solutions, regularly updated
package g0401_0500.s0409_longest_palindrome;
// #Easy #String #Hash_Table #Greedy #Data_Structure_II_Day_6_String #Level_1_Day_5_Greedy
// #2022_07_16_Time_2_ms_(92.90%)_Space_40.5_MB_(95.32%)
import java.util.BitSet;
/**
* 409 - Longest Palindrome\.
*
* Easy
*
* Given a string `s` which consists of lowercase or uppercase letters, return _the length of the **longest palindrome**_ that can be built with those letters.
*
* Letters are **case sensitive** , for example, `"Aa"` is not considered a palindrome here.
*
* **Example 1:**
*
* **Input:** s = "abccccdd"
*
* **Output:** 7
*
* **Explanation:** One longest palindrome that can be built is "dccaccd", whose length is 7.
*
* **Example 2:**
*
* **Input:** s = "a"
*
* **Output:** 1
*
* **Example 3:**
*
* **Input:** s = "bb"
*
* **Output:** 2
*
* **Constraints:**
*
* * `1 <= s.length <= 2000`
* * `s` consists of lowercase **and/or** uppercase English letters only.
**/
public class Solution {
public int longestPalindrome(String s) {
BitSet set = new BitSet(60);
for (char c : s.toCharArray()) {
set.flip(c - 'A');
}
if (set.isEmpty()) {
return s.length();
}
return s.length() - set.cardinality() + 1;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy