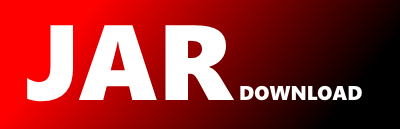
g0501_0600.s0518_coin_change_2.Solution Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of leetcode-in-java21 Show documentation
Show all versions of leetcode-in-java21 Show documentation
Java-based LeetCode algorithm problem solutions, regularly updated
package g0501_0600.s0518_coin_change_2;
// #Medium #Array #Dynamic_Programming #Dynamic_Programming_I_Day_20
// #2022_07_25_Time_4_ms_(84.67%)_Space_42.2_MB_(60.30%)
/**
* 518 - Coin Change 2\.
*
* Medium
*
* You are given an integer array `coins` representing coins of different denominations and an integer `amount` representing a total amount of money.
*
* Return _the number of combinations that make up that amount_. If that amount of money cannot be made up by any combination of the coins, return `0`.
*
* You may assume that you have an infinite number of each kind of coin.
*
* The answer is **guaranteed** to fit into a signed **32-bit** integer.
*
* **Example 1:**
*
* **Input:** amount = 5, coins = [1,2,5]
*
* **Output:** 4
*
* **Explanation:** there are four ways to make up the amount: 5=5 5=2+2+1 5=2+1+1+1 5=1+1+1+1+1
*
* **Example 2:**
*
* **Input:** amount = 3, coins = [2]
*
* **Output:** 0
*
* **Explanation:** the amount of 3 cannot be made up just with coins of 2.
*
* **Example 3:**
*
* **Input:** amount = 10, coins = [10]
*
* **Output:** 1
*
* **Constraints:**
*
* * `1 <= coins.length <= 300`
* * `1 <= coins[i] <= 5000`
* * All the values of `coins` are **unique**.
* * `0 <= amount <= 5000`
**/
public class Solution {
public int change(int amount, int[] coins) {
int[] dp = new int[amount + 1];
dp[0] = 1;
for (int coin : coins) {
for (int i = coin; i <= amount; i++) {
dp[i] += dp[i - coin];
}
}
return dp[amount];
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy