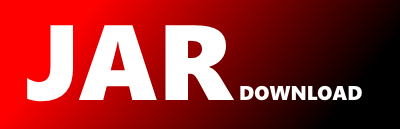
g0501_0600.s0535_encode_and_decode_tinyurl.Codec Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of leetcode-in-java21 Show documentation
Show all versions of leetcode-in-java21 Show documentation
Java-based LeetCode algorithm problem solutions, regularly updated
package g0501_0600.s0535_encode_and_decode_tinyurl;
// #Medium #String #Hash_Table #Design #Hash_Function
// #2022_08_02_Time_2_ms_(89.67%)_Space_42.1_MB_(96.88%)
import java.util.HashMap;
import java.util.Map;
/**
* 535 - Encode and Decode TinyURL\.
*
* Medium
*
* > Note: This is a companion problem to the [System Design](https://leetcode.com/discuss/interview-question/system-design/) problem: [Design TinyURL](https://leetcode.com/discuss/interview-question/124658/Design-a-URL-Shortener-(-TinyURL-)-System/).
*
* TinyURL is a URL shortening service where you enter a URL such as `https://leetcode.com/problems/design-tinyurl` and it returns a short URL such as `http://tinyurl.com/4e9iAk`. Design a class to encode a URL and decode a tiny URL.
*
* There is no restriction on how your encode/decode algorithm should work. You just need to ensure that a URL can be encoded to a tiny URL and the tiny URL can be decoded to the original URL.
*
* Implement the `Solution` class:
*
* * `Solution()` Initializes the object of the system.
* * `String encode(String longUrl)` Returns a tiny URL for the given `longUrl`.
* * `String decode(String shortUrl)` Returns the original long URL for the given `shortUrl`. It is guaranteed that the given `shortUrl` was encoded by the same object.
*
* **Example 1:**
*
* **Input:** url = "https://leetcode.com/problems/design-tinyurl"
*
* **Output:** "https://leetcode.com/problems/design-tinyurl"
*
* **Explanation:**
*
* Solution obj = new Solution();
* string tiny = obj.encode(url); // returns the encoded tiny url.
* string ans = obj.decode(tiny); // returns the original url after deconding it.
*
* **Constraints:**
*
* * 1 <= url.length <= 104
* * `url` is guranteed to be a valid URL.
**/
public class Codec {
private Map map = new HashMap<>();
private int n = 0;
// Encodes a URL to a shortened URL.
public String encode(String longUrl) {
n++;
String ans = "http://tinyurl.com/";
ans += Integer.toString(n);
map.put(ans, longUrl);
return ans;
}
// Decodes a shortened URL to its original URL.
public String decode(String shortUrl) {
return map.get(shortUrl);
}
}
// Your Codec object will be instantiated and called as such:
// Codec codec = new Codec();
// codec.decode(codec.encode(url));
© 2015 - 2025 Weber Informatics LLC | Privacy Policy