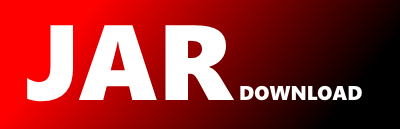
g0501_0600.s0575_distribute_candies.Solution Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of leetcode-in-java21 Show documentation
Show all versions of leetcode-in-java21 Show documentation
Java-based LeetCode algorithm problem solutions, regularly updated
package g0501_0600.s0575_distribute_candies;
// #Easy #Array #Hash_Table #2022_08_10_Time_67_ms_(42.81%)_Space_111.2_MB_(25.92%)
import java.util.HashSet;
import java.util.Set;
/**
* 575 - Distribute Candies\.
*
* Easy
*
* Alice has `n` candies, where the ith
candy is of type `candyType[i]`. Alice noticed that she started to gain weight, so she visited a doctor.
*
* The doctor advised Alice to only eat `n / 2` of the candies she has (`n` is always even). Alice likes her candies very much, and she wants to eat the maximum number of different types of candies while still following the doctor's advice.
*
* Given the integer array `candyType` of length `n`, return _the **maximum** number of different types of candies she can eat if she only eats_ `n / 2` _of them_.
*
* **Example 1:**
*
* **Input:** candyType = [1,1,2,2,3,3]
*
* **Output:** 3
*
* **Explanation:** Alice can only eat 6 / 2 = 3 candies. Since there are only 3 types, she can eat one of each type.
*
* **Example 2:**
*
* **Input:** candyType = [1,1,2,3]
*
* **Output:** 2
*
* **Explanation:** Alice can only eat 4 / 2 = 2 candies. Whether she eats types [1,2], [1,3], or [2,3], she still can only eat 2 different types.
*
* **Example 3:**
*
* **Input:** candyType = [6,6,6,6]
*
* **Output:** 1
*
* **Explanation:** Alice can only eat 4 / 2 = 2 candies. Even though she can eat 2 candies, she only has 1 type.
*
* **Constraints:**
*
* * `n == candyType.length`
* * 2 <= n <= 104
* * `n` is even.
* * -105 <= candyType[i] <= 105
**/
public class Solution {
public int distributeCandies(int[] candyType) {
Set set = new HashSet<>();
for (int i : candyType) {
set.add(i);
}
return Math.min(set.size(), candyType.length / 2);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy