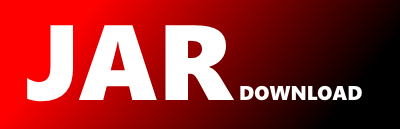
g0501_0600.s0594_longest_harmonious_subsequence.Solution Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of leetcode-in-java21 Show documentation
Show all versions of leetcode-in-java21 Show documentation
Java-based LeetCode algorithm problem solutions, regularly updated
package g0501_0600.s0594_longest_harmonious_subsequence;
// #Easy #Array #Hash_Table #Sorting #2022_08_25_Time_16_ms_(95.95%)_Space_54.5_MB_(74.21%)
import java.util.Arrays;
/**
* 594 - Longest Harmonious Subsequence\.
*
* Easy
*
* We define a harmonious array as an array where the difference between its maximum value and its minimum value is **exactly** `1`.
*
* Given an integer array `nums`, return _the length of its longest harmonious subsequence among all its possible subsequences_.
*
* A **subsequence** of array is a sequence that can be derived from the array by deleting some or no elements without changing the order of the remaining elements.
*
* **Example 1:**
*
* **Input:** nums = [1,3,2,2,5,2,3,7]
*
* **Output:** 5
*
* **Explanation:** The longest harmonious subsequence is [3,2,2,2,3].
*
* **Example 2:**
*
* **Input:** nums = [1,2,3,4]
*
* **Output:** 2
*
* **Example 3:**
*
* **Input:** nums = [1,1,1,1]
*
* **Output:** 0
*
* **Constraints:**
*
* * 1 <= nums.length <= 2 * 104
* * -109 <= nums[i] <= 109
**/
public class Solution {
public int findLHS(int[] nums) {
Arrays.sort(nums);
int max = 0;
int lastN = 0;
int curN = 1;
int cur = nums[0];
for (int i = 1; i < nums.length; i++) {
if (nums[i] > cur) {
if (lastN > 0 && curN > 0 && lastN + curN > max) {
max = lastN + curN;
}
// if diff more than 1, don't count
lastN = nums[i] - cur == 1 ? curN : 0;
curN = 1;
cur = nums[i];
} else {
curN++;
}
}
if (lastN > 0 && curN > 0 && lastN + curN > max) {
max = lastN + curN;
}
return max;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy