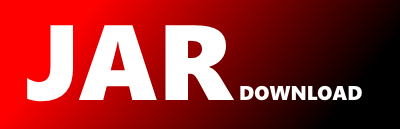
g0601_0700.s0647_palindromic_substrings.Solution Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of leetcode-in-java21 Show documentation
Show all versions of leetcode-in-java21 Show documentation
Java-based LeetCode algorithm problem solutions, regularly updated
package g0601_0700.s0647_palindromic_substrings;
// #Medium #Top_100_Liked_Questions #String #Dynamic_Programming #Big_O_Time_O(n^2)_Space_O(n)
// #2022_03_21_Time_2_ms_(98.77%)_Space_41.7_MB_(75.10%)
/**
* 647 - Palindromic Substrings\.
*
* Medium
*
* Given a string `s`, return _the number of **palindromic substrings** in it_.
*
* A string is a **palindrome** when it reads the same backward as forward.
*
* A **substring** is a contiguous sequence of characters within the string.
*
* **Example 1:**
*
* **Input:** s = "abc"
*
* **Output:** 3
*
* **Explanation:** Three palindromic strings: "a", "b", "c".
*
* **Example 2:**
*
* **Input:** s = "aaa"
*
* **Output:** 6
*
* **Explanation:** Six palindromic strings: "a", "a", "a", "aa", "aa", "aaa".
*
* **Constraints:**
*
* * `1 <= s.length <= 1000`
* * `s` consists of lowercase English letters.
**/
public class Solution {
private void expand(char[] a, int l, int r, int[] res) {
while (l >= 0 && r < a.length) {
if (a[l] != a[r]) {
return;
} else {
res[0]++;
l--;
r++;
}
}
}
public int countSubstrings(String s) {
char[] a = s.toCharArray();
int[] res = {0};
for (int i = 0; i < a.length; i++) {
expand(a, i, i, res);
expand(a, i, i + 1, res);
}
return res[0];
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy