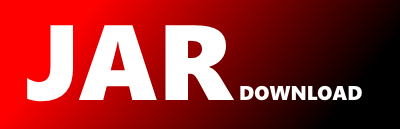
g0801_0900.s0868_binary_gap.Solution Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of leetcode-in-java21 Show documentation
Show all versions of leetcode-in-java21 Show documentation
Java-based LeetCode algorithm problem solutions, regularly updated
package g0801_0900.s0868_binary_gap;
// #Easy #Bit_Manipulation #2022_05_16_Time_1_ms_(82.94%)_Space_41.4_MB_(28.50%)
/**
* 868 - Binary Gap\.
*
* Easy
*
* Given a positive integer `n`, find and return _the **longest distance** between any two **adjacent**_ `1`_'s in the binary representation of_ `n`_. If there are no two adjacent_ `1`_'s, return_ `0`_._
*
* Two `1`'s are **adjacent** if there are only `0`'s separating them (possibly no `0`'s). The **distance** between two `1`'s is the absolute difference between their bit positions. For example, the two `1`'s in `"1001"` have a distance of 3.
*
* **Example 1:**
*
* **Input:** n = 22
*
* **Output:** 2
*
* **Explanation:** 22 in binary is "10110".
*
* The first adjacent pair of 1's is "10110" with a distance of 2.
*
* The second adjacent pair of 1's is "10110" with a distance of 1.
*
* The answer is the largest of these two distances, which is 2.
*
* Note that "10110" is not a valid pair since there is a 1 separating the two 1's underlined.
*
* **Example 2:**
*
* **Input:** n = 8
*
* **Output:** 0
*
* **Explanation:** 8 in binary is "1000". There are not any adjacent pairs of 1's in the binary representation of 8, so we return 0.
*
* **Example 3:**
*
* **Input:** n = 5
*
* **Output:** 2
*
* **Explanation:** 5 in binary is "101".
*
* **Constraints:**
*
* * 1 <= n <= 109
**/
public class Solution {
public int binaryGap(int n) {
int max = 0;
int pos = 0;
int lastPos = -1;
while (n != 0) {
pos++;
if ((n & 1) == 1) {
if (lastPos != -1) {
max = Math.max(max, pos - lastPos);
}
lastPos = pos;
}
n >>= 1;
}
return max;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy