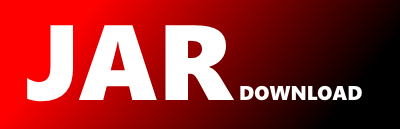
g0801_0900.s0871_minimum_number_of_refueling_stops.Solution Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of leetcode-in-java21 Show documentation
Show all versions of leetcode-in-java21 Show documentation
Java-based LeetCode algorithm problem solutions, regularly updated
package g0801_0900.s0871_minimum_number_of_refueling_stops;
// #Hard #Array #Dynamic_Programming #Greedy #Heap_Priority_Queue
// #2022_03_28_Time_3_ms_(88.52%)_Space_48.8_MB_(60.04%)
import java.util.PriorityQueue;
/**
* 871 - Minimum Number of Refueling Stops\.
*
* Hard
*
* A car travels from a starting position to a destination which is `target` miles east of the starting position.
*
* There are gas stations along the way. The gas stations are represented as an array `stations` where stations[i] = [positioni, fueli]
indicates that the ith
gas station is positioni
miles east of the starting position and has fueli
liters of gas.
*
* The car starts with an infinite tank of gas, which initially has `startFuel` liters of fuel in it. It uses one liter of gas per one mile that it drives. When the car reaches a gas station, it may stop and refuel, transferring all the gas from the station into the car.
*
* Return _the minimum number of refueling stops the car must make in order to reach its destination_. If it cannot reach the destination, return `-1`.
*
* Note that if the car reaches a gas station with `0` fuel left, the car can still refuel there. If the car reaches the destination with `0` fuel left, it is still considered to have arrived.
*
* **Example 1:**
*
* **Input:** target = 1, startFuel = 1, stations = []
*
* **Output:** 0
*
* **Explanation:** We can reach the target without refueling.
*
* **Example 2:**
*
* **Input:** target = 100, startFuel = 1, stations = \[\[10,100]]
*
* **Output:** -1
*
* **Explanation:** We can not reach the target (or even the first gas station).
*
* **Example 3:**
*
* **Input:** target = 100, startFuel = 10, stations = \[\[10,60],[20,30],[30,30],[60,40]]
*
* **Output:** 2
*
* **Explanation:** We start with 10 liters of fuel. We drive to position 10, expending 10 liters of fuel.
*
* We refuel from 0 liters to 60 liters of gas.
*
* Then, we drive from position 10 to position 60 (expending 50 liters of fuel),
*
* and refuel from 10 liters to 50 liters of gas. We then drive to and reach the target.
*
* We made 2 refueling stops along the way, so we return 2.
*
* **Constraints:**
*
* * 1 <= target, startFuel <= 109
* * `0 <= stations.length <= 500`
* * 0 <= positioni <= positioni+1 < target
* * 1 <= fueli < 109
**/
public class Solution {
public int minRefuelStops(int target, int startFuel, int[][] stations) {
if (startFuel >= target) {
return 0;
} else if (stations == null || stations.length == 0) {
return -1;
}
PriorityQueue pq = new PriorityQueue<>((a, b) -> b[1] - a[1]);
int start = 0;
int end = stations.length;
int currentFuel = startFuel;
int stops = 0;
while (currentFuel < target) {
while (start < end && currentFuel >= stations[start][0]) {
pq.add(stations[start++]);
}
if (pq.isEmpty()) {
return -1;
}
int[] current = pq.poll();
currentFuel += current[1];
stops++;
}
return stops;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy