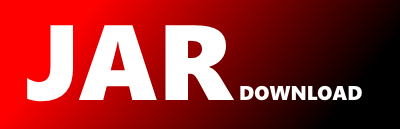
g0801_0900.s0875_koko_eating_bananas.Solution Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of leetcode-in-java21 Show documentation
Show all versions of leetcode-in-java21 Show documentation
Java-based LeetCode algorithm problem solutions, regularly updated
package g0801_0900.s0875_koko_eating_bananas;
// #Medium #Array #Binary_Search #Binary_Search_II_Day_4
// #2022_03_28_Time_15_ms_(91.32%)_Space_55_MB_(6.01%)
/**
* 875 - Koko Eating Bananas\.
*
* Medium
*
* Koko loves to eat bananas. There are `n` piles of bananas, the ith
pile has `piles[i]` bananas. The guards have gone and will come back in `h` hours.
*
* Koko can decide her bananas-per-hour eating speed of `k`. Each hour, she chooses some pile of bananas and eats `k` bananas from that pile. If the pile has less than `k` bananas, she eats all of them instead and will not eat any more bananas during this hour.
*
* Koko likes to eat slowly but still wants to finish eating all the bananas before the guards return.
*
* Return _the minimum integer_ `k` _such that she can eat all the bananas within_ `h` _hours_.
*
* **Example 1:**
*
* **Input:** piles = [3,6,7,11], h = 8
*
* **Output:** 4
*
* **Example 2:**
*
* **Input:** piles = [30,11,23,4,20], h = 5
*
* **Output:** 30
*
* **Example 3:**
*
* **Input:** piles = [30,11,23,4,20], h = 6
*
* **Output:** 23
*
* **Constraints:**
*
* * 1 <= piles.length <= 104
* * piles.length <= h <= 109
* * 1 <= piles[i] <= 109
**/
public class Solution {
public int minEatingSpeed(int[] piles, int h) {
int maxP = piles[0];
long sumP = 0L;
for (int pile : piles) {
maxP = Math.max(maxP, pile);
sumP += pile;
}
// binary search
int low = (int) ((sumP - 1) / h + 1);
int high = maxP;
while (low < high) {
int mid = low + (high - low) / 2;
if (isPossible(piles, mid, h)) {
high = mid;
} else {
low = mid + 1;
}
}
return low;
}
private boolean isPossible(int[] piles, int k, int h) {
int sum = 0;
for (int pile : piles) {
sum += (pile - 1) / k + 1;
}
return sum <= h;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy