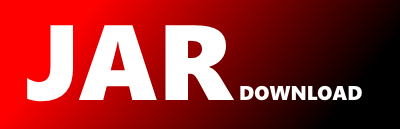
g0901_1000.s0908_smallest_range_i.Solution Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of leetcode-in-java21 Show documentation
Show all versions of leetcode-in-java21 Show documentation
Java-based LeetCode algorithm problem solutions, regularly updated
package g0901_1000.s0908_smallest_range_i;
// #Easy #Array #Math #2022_03_28_Time_2_ms_(88.84%)_Space_41.9_MB_(99.76%)
/**
* 908 - Smallest Range I\.
*
* Easy
*
* You are given an integer array `nums` and an integer `k`.
*
* In one operation, you can choose any index `i` where `0 <= i < nums.length` and change `nums[i]` to `nums[i] + x` where `x` is an integer from the range `[-k, k]`. You can apply this operation **at most once** for each index `i`.
*
* The **score** of `nums` is the difference between the maximum and minimum elements in `nums`.
*
* Return _the minimum **score** of_ `nums` _after applying the mentioned operation at most once for each index in it_.
*
* **Example 1:**
*
* **Input:** nums = [1], k = 0
*
* **Output:** 0
*
* **Explanation:** The score is max(nums) - min(nums) = 1 - 1 = 0.
*
* **Example 2:**
*
* **Input:** nums = [0,10], k = 2
*
* **Output:** 6
*
* **Explanation:** Change nums to be [2, 8]. The score is max(nums) - min(nums) = 8 - 2 = 6.
*
* **Example 3:**
*
* **Input:** nums = [1,3,6], k = 3
*
* **Output:** 0
*
* **Explanation:** Change nums to be [4, 4, 4]. The score is max(nums) - min(nums) = 4 - 4 = 0.
*
* **Constraints:**
*
* * 1 <= nums.length <= 104
* * 0 <= nums[i] <= 104
* * 0 <= k <= 104
**/
public class Solution {
public int smallestRangeI(int[] nums, int k) {
int min = Integer.MAX_VALUE;
int max = Integer.MIN_VALUE;
for (int num : nums) {
min = Math.min(min, num);
max = Math.max(max, num);
}
if (min + k >= max - k) {
return 0;
}
return (max - k) - (min + k);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy