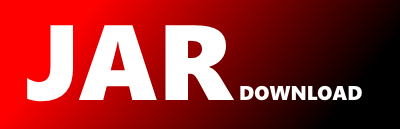
g0901_1000.s0953_verifying_an_alien_dictionary.Solution Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of leetcode-in-java21 Show documentation
Show all versions of leetcode-in-java21 Show documentation
Java-based LeetCode algorithm problem solutions, regularly updated
package g0901_1000.s0953_verifying_an_alien_dictionary;
// #Easy #Array #String #Hash_Table #Programming_Skills_I_Day_9_String
// #2022_12_26_Time_0_ms_(100.00%)_Space_40.7_MB_(94.41%)
/**
* 953 - Verifying an Alien Dictionary\.
*
* Easy
*
* In an alien language, surprisingly, they also use English lowercase letters, but possibly in a different `order`. The `order` of the alphabet is some permutation of lowercase letters.
*
* Given a sequence of `words` written in the alien language, and the `order` of the alphabet, return `true` if and only if the given `words` are sorted lexicographically in this alien language.
*
* **Example 1:**
*
* **Input:** words = ["hello","leetcode"], order = "hlabcdefgijkmnopqrstuvwxyz"
*
* **Output:** true
*
* **Explanation:** As 'h' comes before 'l' in this language, then the sequence is sorted.
*
* **Example 2:**
*
* **Input:** words = ["word","world","row"], order = "worldabcefghijkmnpqstuvxyz"
*
* **Output:** false
*
* **Explanation:** As 'd' comes after 'l' in this language, then words[0] > words[1], hence the sequence is unsorted.
*
* **Example 3:**
*
* **Input:** words = ["apple","app"], order = "abcdefghijklmnopqrstuvwxyz"
*
* **Output:** false
*
* **Explanation:** The first three characters "app" match, and the second string is shorter (in size.) According to lexicographical rules "apple" > "app", because 'l' > '∅', where '∅' is defined as the blank character which is less than any other character ([More info](https://en.wikipedia.org/wiki/Lexicographical_order)).
*
* **Constraints:**
*
* * `1 <= words.length <= 100`
* * `1 <= words[i].length <= 20`
* * `order.length == 26`
* * All characters in `words[i]` and `order` are English lowercase letters.
**/
public class Solution {
public boolean isAlienSorted(String[] words, String order) {
int[] map = new int[26];
for (int i = 0; i < order.length(); i++) {
map[order.charAt(i) - 'a'] = i;
}
for (int i = 0; i < words.length - 1; i++) {
if (!isSmaller(words[i], words[i + 1], map)) {
return false;
}
}
return true;
}
private boolean isSmaller(String str1, String str2, int[] map) {
int len1 = str1.length();
int len2 = str2.length();
int minLength = Math.min(len1, len2);
for (int i = 0; i < minLength; i++) {
if (map[str1.charAt(i) - 'a'] > map[str2.charAt(i) - 'a']) {
return false;
} else if (map[str1.charAt(i) - 'a'] < map[str2.charAt(i) - 'a']) {
return true;
}
}
return len1 <= len2;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy