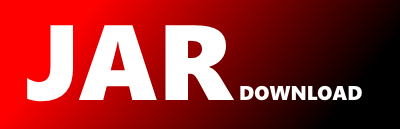
g1001_1100.s1021_remove_outermost_parentheses.Solution Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of leetcode-in-java21 Show documentation
Show all versions of leetcode-in-java21 Show documentation
Java-based LeetCode algorithm problem solutions, regularly updated
package g1001_1100.s1021_remove_outermost_parentheses;
// #Easy #String #Stack #2022_02_25_Time_4_ms_(75.39%)_Space_42.3_MB_(50.45%)
import java.util.ArrayList;
import java.util.List;
/**
* 1021 - Remove Outermost Parentheses\.
*
* Easy
*
* A valid parentheses string is either empty `""`, `"(" + A + ")"`, or `A + B`, where `A` and `B` are valid parentheses strings, and `+` represents string concatenation.
*
* * For example, `""`, `"()"`, `"(())()"`, and `"(()(()))"` are all valid parentheses strings.
*
* A valid parentheses string `s` is primitive if it is nonempty, and there does not exist a way to split it into `s = A + B`, with `A` and `B` nonempty valid parentheses strings.
*
* Given a valid parentheses string `s`, consider its primitive decomposition: s = P1 + P2 + ... + Pk
, where Pi
are primitive valid parentheses strings.
*
* Return `s` _after removing the outermost parentheses of every primitive string in the primitive decomposition of_ `s`.
*
* **Example 1:**
*
* **Input:** s = "(()())(())"
*
* **Output:** "()()()"
*
* **Explanation:**
*
* The input string is "(()())(())", with primitive decomposition "(()())" + "(())".
*
* After removing outer parentheses of each part, this is "()()" + "()" = "()()()".
*
* **Example 2:**
*
* **Input:** s = "(()())(())(()(()))"
*
* **Output:** "()()()()(())"
*
* **Explanation:**
*
* The input string is "(()())(())(()(()))", with primitive decomposition "(()())" + "(())" + "(()(()))".
*
* After removing outer parentheses of each part, this is "()()" + "()" + "()(())" = "()()()()(())".
*
* **Example 3:**
*
* **Input:** s = "()()"
*
* **Output:** ""
*
* **Explanation:**
*
* The input string is "()()", with primitive decomposition "()" + "()".
*
* After removing outer parentheses of each part, this is "" + "" = "".
*
* **Constraints:**
*
* * 1 <= s.length <= 105
* * `s[i]` is either `'('` or `')'`.
* * `s` is a valid parentheses string.
**/
public class Solution {
public String removeOuterParentheses(String s) {
List primitives = new ArrayList<>();
int i = 1;
while (i < s.length()) {
int initialI = i - 1;
int left = 1;
while (i < s.length() && left > 0) {
if (s.charAt(i) == '(') {
left++;
} else {
left--;
}
i++;
}
primitives.add(s.substring(initialI, i));
i++;
}
StringBuilder sb = new StringBuilder();
for (String primitive : primitives) {
sb.append(primitive, 1, primitive.length() - 1);
}
return sb.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy