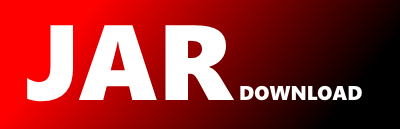
g1301_1400.s1323_maximum_69_number.Solution Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of leetcode-in-java21 Show documentation
Show all versions of leetcode-in-java21 Show documentation
Java-based LeetCode algorithm problem solutions, regularly updated
package g1301_1400.s1323_maximum_69_number;
// #Easy #Math #Greedy #2022_03_19_Time_3_ms_(32.03%)_Space_39.5_MB_(67.69%)
import java.util.stream.IntStream;
/**
* 1323 - Maximum 69 Number\.
*
* Easy
*
* You are given a positive integer `num` consisting only of digits `6` and `9`.
*
* Return _the maximum number you can get by changing **at most** one digit (_`6` _becomes_ `9`_, and_ `9` _becomes_ `6`_)_.
*
* **Example 1:**
*
* **Input:** num = 9669
*
* **Output:** 9969
*
* **Explanation:**
*
* Changing the first digit results in 6669.
*
* Changing the second digit results in 9969.
*
* Changing the third digit results in 9699.
*
* Changing the fourth digit results in 9666.
*
* The maximum number is 9969.
*
* **Example 2:**
*
* **Input:** num = 9996
*
* **Output:** 9999
*
* **Explanation:** Changing the last digit 6 to 9 results in the maximum number.
*
* **Example 3:**
*
* **Input:** num = 9999
*
* **Output:** 9999
*
* **Explanation:** It is better not to apply any change.
*
* **Constraints:**
*
* * 1 <= num <= 104
* * `num` consists of only `6` and `9` digits.
**/
public class Solution {
public int maximum69Number(int num) {
char[] chars = Integer.toString(num).toCharArray();
IntStream.range(0, chars.length)
.filter(i -> chars[i] == '6')
.findFirst()
.ifPresent(i -> chars[i] = '9');
return Integer.parseInt(new String(chars));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy