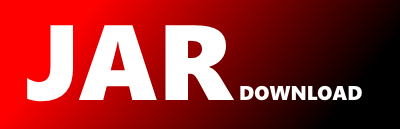
g1401_1500.s1410_html_entity_parser.Solution Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of leetcode-in-java21 Show documentation
Show all versions of leetcode-in-java21 Show documentation
Java-based LeetCode algorithm problem solutions, regularly updated
package g1401_1500.s1410_html_entity_parser;
// #Medium #String #Hash_Table #2022_03_27_Time_19_ms_(98.92%)_Space_42.6_MB_(100.00%)
import java.util.HashMap;
import java.util.Map;
/**
* 1410 - HTML Entity Parser\.
*
* Medium
*
* **HTML entity parser** is the parser that takes HTML code as input and replace all the entities of the special characters by the characters itself.
*
* The special characters and their entities for HTML are:
*
* * **Quotation Mark:** the entity is `"` and symbol character is `"`.
* * **Single Quote Mark:** the entity is `'` and symbol character is `'`.
* * **Ampersand:** the entity is `&` and symbol character is `&`.
* * **Greater Than Sign:** the entity is `>` and symbol character is `>`.
* * **Less Than Sign:** the entity is `<` and symbol character is `<`.
* * **Slash:** the entity is `⁄` and symbol character is `/`.
*
* Given the input `text` string to the HTML parser, you have to implement the entity parser.
*
* Return _the text after replacing the entities by the special characters_.
*
* **Example 1:**
*
* **Input:** text = "& is an HTML entity but &ambassador; is not."
*
* **Output:** "& is an HTML entity but &ambassador; is not."
*
* **Explanation:** The parser will replace the & entity by &
*
* **Example 2:**
*
* **Input:** text = "and I quote: "...""
*
* **Output:** "and I quote: \\"...\\""
*
* **Constraints:**
*
* * 1 <= text.length <= 105
* * The string may contain any possible characters out of all the 256 ASCII characters.
**/
public class Solution {
public String entityParser(String text) {
Map map = new HashMap<>();
map.put(""", "\"");
map.put("'", "'");
map.put("&", "&");
map.put(">", ">");
map.put("<", "<");
map.put("⁄", "/");
int n = text.length();
StringBuilder sb = new StringBuilder();
int i = 0;
while (i < n) {
char c = text.charAt(i);
if (c == '&') {
int index = text.indexOf(";", i);
if (index >= 0) {
String pattern = text.substring(i, index + 1);
if (map.containsKey(pattern)) {
sb.append(map.get(pattern));
i += pattern.length();
continue;
}
}
}
sb.append(c);
i++;
}
return sb.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy