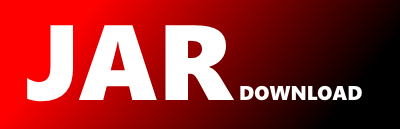
g1501_1600.s1528_shuffle_string.Solution Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of leetcode-in-java21 Show documentation
Show all versions of leetcode-in-java21 Show documentation
Java-based LeetCode algorithm problem solutions, regularly updated
package g1501_1600.s1528_shuffle_string;
// #Easy #Array #String #2022_04_09_Time_2_ms_(54.77%)_Space_45_MB_(34.67%)
/**
* 1528 - Shuffle String\.
*
* Easy
*
* You are given a string `s` and an integer array `indices` of the **same length**. The string `s` will be shuffled such that the character at the ith
position moves to `indices[i]` in the shuffled string.
*
* Return _the shuffled string_.
*
* **Example 1:**
*
* 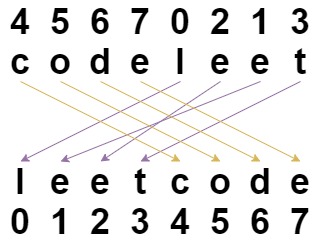
*
* **Input:** s = "codeleet", `indices` = [4,5,6,7,0,2,1,3]
*
* **Output:** "leetcode"
*
* **Explanation:** As shown, "codeleet" becomes "leetcode" after shuffling.
*
* **Example 2:**
*
* **Input:** s = "abc", `indices` = [0,1,2]
*
* **Output:** "abc"
*
* **Explanation:** After shuffling, each character remains in its position.
*
* **Constraints:**
*
* * `s.length == indices.length == n`
* * `1 <= n <= 100`
* * `s` consists of only lowercase English letters.
* * `0 <= indices[i] < n`
* * All values of `indices` are **unique**.
**/
public class Solution {
public String restoreString(String s, int[] indices) {
char[] c = new char[s.length()];
for (int i = 0; i < s.length(); i++) {
int index = findIndex(indices, i);
c[i] = s.charAt(index);
}
return new String(c);
}
private static int findIndex(int[] indices, int i) {
for (int j = 0; j < indices.length; j++) {
if (indices[j] == i) {
return j;
}
}
return 0;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy