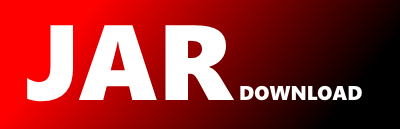
g1701_1800.s1768_merge_strings_alternately.Solution Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of leetcode-in-java21 Show documentation
Show all versions of leetcode-in-java21 Show documentation
Java-based LeetCode algorithm problem solutions, regularly updated
package g1701_1800.s1768_merge_strings_alternately;
// #Easy #String #Two_Pointers #Programming_Skills_I_Day_8_String
// #2022_04_27_Time_1_ms_(86.26%)_Space_41.7_MB_(79.68%)
/**
* 1768 - Merge Strings Alternately\.
*
* Easy
*
* You are given two strings `word1` and `word2`. Merge the strings by adding letters in alternating order, starting with `word1`. If a string is longer than the other, append the additional letters onto the end of the merged string.
*
* Return _the merged string._
*
* **Example 1:**
*
* **Input:** word1 = "abc", word2 = "pqr"
*
* **Output:** "apbqcr"
*
* **Explanation:** The merged string will be merged as so:
*
* word1: a b c
*
* word2: p q r
*
* merged: a p b q c r
*
* **Example 2:**
*
* **Input:** word1 = "ab", word2 = "pqrs"
*
* **Output:** "apbqrs"
*
* **Explanation:** Notice that as word2 is longer, "rs" is appended to the end.
*
* word1: a b
*
* word2: p q r s
*
* merged: a p b q r s
*
* **Example 3:**
*
* **Input:** word1 = "abcd", word2 = "pq"
*
* **Output:** "apbqcd"
*
* **Explanation:** Notice that as word1 is longer, "cd" is appended to the end.
*
* word1: a b c d
*
* word2: p q
*
* merged: a p b q c d
*
* **Constraints:**
*
* * `1 <= word1.length, word2.length <= 100`
* * `word1` and `word2` consist of lowercase English letters.
**/
public class Solution {
public String mergeAlternately(String word1, String word2) {
int size1 = word1.length();
int size2 = word2.length();
int min = Math.min(size1, size2);
StringBuilder sb = new StringBuilder();
for (int i = 0; i < min; i++) {
sb.append(word1.charAt(i));
sb.append(word2.charAt(i));
}
if (min == size1) {
sb.append(word2, size1, size2);
}
if (min == size2) {
sb.append(word1, size2, size1);
}
return sb.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy