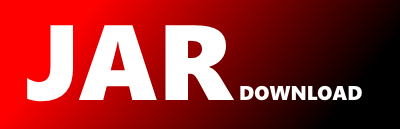
g1701_1800.s1800_maximum_ascending_subarray_sum.Solution Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of leetcode-in-java21 Show documentation
Show all versions of leetcode-in-java21 Show documentation
Java-based LeetCode algorithm problem solutions, regularly updated
package g1701_1800.s1800_maximum_ascending_subarray_sum;
// #Easy #Array #2022_04_19_Time_0_ms_(100.00%)_Space_42.1_MB_(22.29%)
/**
* 1800 - Maximum Ascending Subarray Sum\.
*
* Easy
*
* Given an array of positive integers `nums`, return the _maximum possible sum of an **ascending** subarray in_ `nums`.
*
* A subarray is defined as a contiguous sequence of numbers in an array.
*
* A subarray [numsl, numsl+1, ..., numsr-1, numsr]
is **ascending** if for all `i` where `l <= i < r`, numsi < numsi+1
. Note that a subarray of size `1` is **ascending**.
*
* **Example 1:**
*
* **Input:** nums = [10,20,30,5,10,50]
*
* **Output:** 65
*
* **Explanation:** [5,10,50] is the ascending subarray with the maximum sum of 65.
*
* **Example 2:**
*
* **Input:** nums = [10,20,30,40,50]
*
* **Output:** 150
*
* **Explanation:** [10,20,30,40,50] is the ascending subarray with the maximum sum of 150.
*
* **Example 3:**
*
* **Input:** nums = [12,17,15,13,10,11,12]
*
* **Output:** 33
*
* **Explanation:** [10,11,12] is the ascending subarray with the maximum sum of 33.
*
* **Constraints:**
*
* * `1 <= nums.length <= 100`
* * `1 <= nums[i] <= 100`
**/
public class Solution {
public int maxAscendingSum(int[] nums) {
int maxSum = nums[0];
int i = 0;
int j = i + 1;
while (i < nums.length - 1 && j < nums.length) {
int sum = nums[j - 1];
while (j < nums.length && nums[j] - nums[j - 1] > 0) {
sum += nums[j];
j++;
}
i = j;
maxSum = Math.max(maxSum, sum);
j++;
}
return maxSum;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy