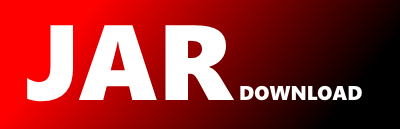
g1801_1900.s1845_seat_reservation_manager.SeatManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of leetcode-in-java21 Show documentation
Show all versions of leetcode-in-java21 Show documentation
Java-based LeetCode algorithm problem solutions, regularly updated
package g1801_1900.s1845_seat_reservation_manager;
// #Medium #Design #Heap_Priority_Queue #Programming_Skills_II_Day_17
// #2022_05_07_Time_47_ms_(87.63%)_Space_107.1_MB_(45.03%)
import java.util.PriorityQueue;
import java.util.Queue;
/**
* 1845 - Seat Reservation Manager\.
*
* Medium
*
* Design a system that manages the reservation state of `n` seats that are numbered from `1` to `n`.
*
* Implement the `SeatManager` class:
*
* * `SeatManager(int n)` Initializes a `SeatManager` object that will manage `n` seats numbered from `1` to `n`. All seats are initially available.
* * `int reserve()` Fetches the **smallest-numbered** unreserved seat, reserves it, and returns its number.
* * `void unreserve(int seatNumber)` Unreserves the seat with the given `seatNumber`.
*
* **Example 1:**
*
* **Input** ["SeatManager", "reserve", "reserve", "unreserve", "reserve", "reserve", "reserve", "reserve", "unreserve"] [[5], [], [], [2], [], [], [], [], [5]]
*
* **Output:** [null, 1, 2, null, 2, 3, 4, 5, null]
*
* **Explanation:**
*
* SeatManager seatManager = new SeatManager(5); // Initializes a SeatManager with 5 seats.
*
* seatManager.reserve(); // All seats are available, so return the lowest numbered seat, which is 1.
*
* seatManager.reserve(); // The available seats are [2,3,4,5], so return the lowest of them, which is 2.
*
* seatManager.unreserve(2); // Unreserve seat 2, so now the available seats are [2,3,4,5].
*
* seatManager.reserve(); // The available seats are [2,3,4,5], so return the lowest of them, which is 2.
*
* seatManager.reserve(); // The available seats are [3,4,5], so return the lowest of them, which is 3.
*
* seatManager.reserve(); // The available seats are [4,5], so return the lowest of them, which is 4.
*
* seatManager.reserve(); // The only available seat is seat 5, so return 5.
*
* seatManager.unreserve(5); // Unreserve seat 5, so now the available seats are [5].
*
* **Constraints:**
*
* * 1 <= n <= 105
* * `1 <= seatNumber <= n`
* * For each call to `reserve`, it is guaranteed that there will be at least one unreserved seat.
* * For each call to `unreserve`, it is guaranteed that `seatNumber` will be reserved.
* * At most 105
calls **in total** will be made to `reserve` and `unreserve`.
**/
@SuppressWarnings("java:S1172")
public class SeatManager {
private final Queue seats;
private int smallest;
public SeatManager(int n) {
seats = new PriorityQueue<>();
smallest = 0;
}
public int reserve() {
return seats.isEmpty() ? ++smallest : seats.poll();
}
public void unreserve(int seatNumber) {
seats.offer(seatNumber);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy