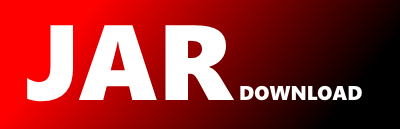
g1801_1900.s1861_rotating_the_box.Solution Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of leetcode-in-java21 Show documentation
Show all versions of leetcode-in-java21 Show documentation
Java-based LeetCode algorithm problem solutions, regularly updated
package g1801_1900.s1861_rotating_the_box;
// #Medium #Array #Matrix #Two_Pointers #2022_05_09_Time_8_ms_(92.84%)_Space_145.6_MB_(5.03%)
/**
* 1861 - Rotating the Box\.
*
* Medium
*
* You are given an `m x n` matrix of characters `box` representing a side-view of a box. Each cell of the box is one of the following:
*
* * A stone `'#'`
* * A stationary obstacle `'*'`
* * Empty `'.'`
*
* The box is rotated **90 degrees clockwise** , causing some of the stones to fall due to gravity. Each stone falls down until it lands on an obstacle, another stone, or the bottom of the box. Gravity **does not** affect the obstacles' positions, and the inertia from the box's rotation **does not** affect the stones' horizontal positions.
*
* It is **guaranteed** that each stone in `box` rests on an obstacle, another stone, or the bottom of the box.
*
* Return _an_ `n x m` _matrix representing the box after the rotation described above_.
*
* **Example 1:**
*
* 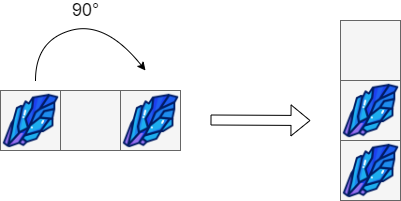
*
* **Input:**
*
* box = \[\["#",".","#"]]
*
* **Output:**
*
* [["."],
* ["#"],
* ["#"]]
*
* **Example 2:**
*
* 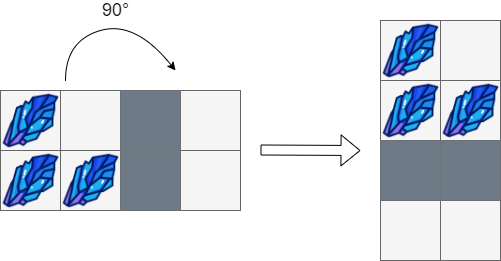
*
* **Input:**
*
* box = \[\["#",".","*","."],
* ["#","#","*","."]]
*
* **Output:**
*
* [["#","."],
* ["#","#"],
* ["*","*"],
* [".","."]]
*
* **Example 3:**
*
* 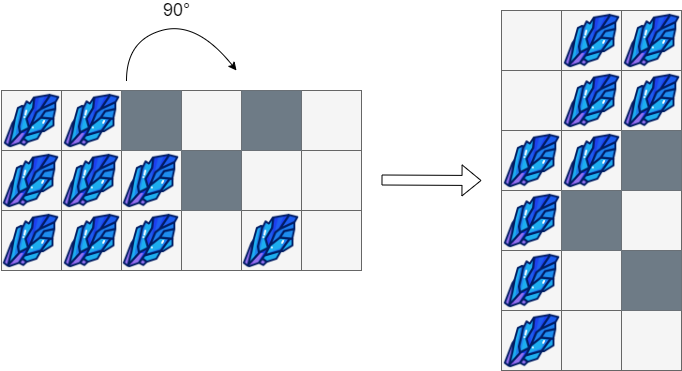
*
* **Input:**
*
* box = \[\["#","#","*",".","*","."],
* ["#","#","#","*",".","."],
* ["#","#","#",".","#","."]]
*
* **Output:**
*
* [[".","#","#"],
* [".","#","#"],
* ["#","#","*"],
* ["#","*","."],
* ["#",".","*"],
* ["#",".","."]]
*
* **Constraints:**
*
* * `m == box.length`
* * `n == box[i].length`
* * `1 <= m, n <= 500`
* * `box[i][j]` is either `'#'`, `'*'`, or `'.'`.
**/
public class Solution {
public char[][] rotateTheBox(char[][] box) {
int n = box.length;
int m = box[0].length;
char[][] result = new char[m][n];
for (int i = 0; i < n; i++) {
int j = m - 1;
int idx = m - 1;
while (j >= 0) {
if (box[i][j] == '#') {
result[j--][n - i - 1] = '.';
result[idx--][n - i - 1] = '#';
} else {
char c = box[i][j];
result[j--][n - i - 1] = c;
if (c == '*') {
idx = j;
}
}
}
}
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy