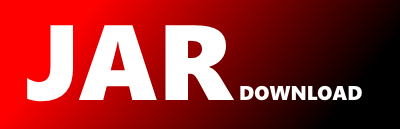
g2001_2100.s2069_walking_robot_simulation_ii.Robot Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of leetcode-in-java21 Show documentation
Show all versions of leetcode-in-java21 Show documentation
Java-based LeetCode algorithm problem solutions, regularly updated
package g2001_2100.s2069_walking_robot_simulation_ii;
// #Medium #Design #Simulation #2022_05_30_Time_110_ms_(56.14%)_Space_95.2_MB_(53.51%)
/**
* 2069 - Walking Robot Simulation II\.
*
* Medium
*
* A `width x height` grid is on an XY-plane with the **bottom-left** cell at `(0, 0)` and the **top-right** cell at `(width - 1, height - 1)`. The grid is aligned with the four cardinal directions (`"North"`, `"East"`, `"South"`, and `"West"`). A robot is **initially** at cell `(0, 0)` facing direction `"East"`.
*
* The robot can be instructed to move for a specific number of **steps**. For each step, it does the following.
*
* 1. Attempts to move **forward one** cell in the direction it is facing.
* 2. If the cell the robot is **moving to** is **out of bounds** , the robot instead **turns** 90 degrees **counterclockwise** and retries the step.
*
* After the robot finishes moving the number of steps required, it stops and awaits the next instruction.
*
* Implement the `Robot` class:
*
* * `Robot(int width, int height)` Initializes the `width x height` grid with the robot at `(0, 0)` facing `"East"`.
* * `void step(int num)` Instructs the robot to move forward `num` steps.
* * `int[] getPos()` Returns the current cell the robot is at, as an array of length 2, `[x, y]`.
* * `String getDir()` Returns the current direction of the robot, `"North"`, `"East"`, `"South"`, or `"West"`.
*
* **Example 1:**
*
* 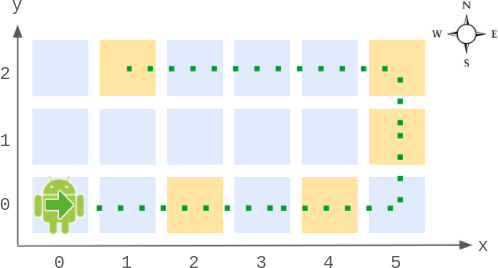
*
* **Input**
*
* ["Robot", "step", "step", "getPos", "getDir", "step", "step", "step", "getPos", "getDir"]
*
* [[6, 3], [2], [2], [], [], [2], [1], [4], [], []]
*
* **Output:**
*
* [null, null, null, [4, 0], "East", null, null, null, [1, 2], "West"]
*
* **Explanation:**
*
* Robot robot = new Robot(6, 3); // Initialize the grid and the robot at (0, 0) facing East.
* robot.step(2); // It moves two steps East to (2, 0), and faces East.
* robot.step(2); // It moves two steps East to (4, 0), and faces East.
* robot.getPos(); // return [4, 0]
* robot.getDir(); // return "East"
* robot.step(2); // It moves one step East to (5, 0), and faces East.
* // Moving the next step East would be out of bounds, so it turns and faces North.
* // Then, it moves one step North to (5, 1), and faces North.
* robot.step(1); // It moves one step North to (5, 2), and faces North (not West).
* robot.step(4); // Moving the next step North would be out of bounds, so it turns and faces West.
* // Then, it moves four steps West to (1, 2), and faces West.
* robot.getPos(); // return [1, 2]
* robot.getDir(); // return "West"
*
* **Constraints:**
*
* * `2 <= width, height <= 100`
* * 1 <= num <= 105
* * At most 104
calls **in total** will be made to `step`, `getPos`, and `getDir`.
**/
public class Robot {
private int p;
private int w;
private int h;
public Robot(int width, int height) {
w = width - 1;
h = height - 1;
p = 0;
}
public void step(int num) {
p += num;
}
public int[] getPos() {
int remain = p % (2 * (w + h));
if (remain <= w) {
return new int[] {remain, 0};
}
remain -= w;
if (remain <= h) {
return new int[] {w, remain};
}
remain -= h;
if (remain <= w) {
return new int[] {w - remain, h};
}
remain -= w;
return new int[] {0, h - remain};
}
public String getDir() {
int[] pos = getPos();
if (p == 0 || pos[1] == 0 && pos[0] > 0) {
return "East";
} else if (pos[0] == w && pos[1] > 0) {
return "North";
} else if (pos[1] == h && pos[0] < w) {
return "West";
} else {
return "South";
}
}
}
/*
* Your Robot object will be instantiated and called as such:
* Robot obj = new Robot(width, height);
* obj.step(num);
* int[] param_2 = obj.getPos();
* String param_3 = obj.getDir();
*/
© 2015 - 2025 Weber Informatics LLC | Privacy Policy