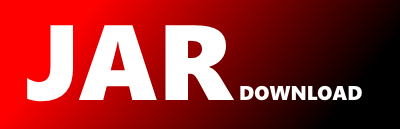
g2101_2200.s2129_capitalize_the_title.Solution Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of leetcode-in-java21 Show documentation
Show all versions of leetcode-in-java21 Show documentation
Java-based LeetCode algorithm problem solutions, regularly updated
package g2101_2200.s2129_capitalize_the_title;
// #Easy #String #2022_06_03_Time_2_ms_(94.22%)_Space_42.5_MB_(71.58%)
/**
* 2129 - Capitalize the Title\.
*
* Easy
*
* You are given a string `title` consisting of one or more words separated by a single space, where each word consists of English letters. **Capitalize** the string by changing the capitalization of each word such that:
*
* * If the length of the word is `1` or `2` letters, change all letters to lowercase.
* * Otherwise, change the first letter to uppercase and the remaining letters to lowercase.
*
* Return _the **capitalized**_ `title`.
*
* **Example 1:**
*
* **Input:** title = "capiTalIze tHe titLe"
*
* **Output:** "Capitalize The Title"
*
* **Explanation:** Since all the words have a length of at least 3, the first letter of each word is uppercase, and the remaining letters are lowercase.
*
* **Example 2:**
*
* **Input:** title = "First leTTeR of EACH Word"
*
* **Output:** "First Letter of Each Word"
*
* **Explanation:**
*
* The word "of" has length 2, so it is all lowercase.
*
* The remaining words have a length of at least 3, so the first letter of each remaining word is uppercase, and the remaining letters are lowercase.
*
* **Example 3:**
*
* **Input:** title = "i lOve leetcode"
*
* **Output:** "i Love Leetcode"
*
* **Explanation:**
*
* The word "i" has length 1, so it is lowercase.
*
* The remaining words have a length of at least 3, so the first letter of each remaining word is uppercase, and the remaining letters are lowercase.
*
* **Constraints:**
*
* * `1 <= title.length <= 100`
* * `title` consists of words separated by a single space without any leading or trailing spaces.
* * Each word consists of uppercase and lowercase English letters and is **non-empty**.
**/
public class Solution {
public String capitalizeTitle(String title) {
StringBuilder sb = new StringBuilder();
int i = 0;
int j = 0;
while (i < title.length()) {
while (j < title.length() && title.charAt(j) != ' ') {
sb.append(Character.toLowerCase(title.charAt(j)));
j++;
}
int len = j - i;
if (len > 2) {
sb.setCharAt(i, Character.toUpperCase(title.charAt(i)));
}
if (j == title.length()) {
break;
}
sb.append(title.charAt(j));
i = ++j;
}
return sb.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy