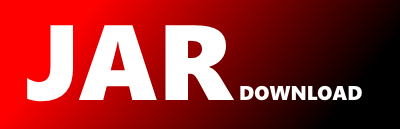
g2301_2400.s2325_decode_the_message.Solution Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of leetcode-in-java21 Show documentation
Show all versions of leetcode-in-java21 Show documentation
Java-based LeetCode algorithm problem solutions, regularly updated
package g2301_2400.s2325_decode_the_message;
// #Easy #String #Hash_Table #2022_07_03_Time_7_ms_(42.86%)_Space_42.6_MB_(57.14%)
import java.util.HashMap;
import java.util.Map;
/**
* 2325 - Decode the Message\.
*
* Easy
*
* You are given the strings `key` and `message`, which represent a cipher key and a secret message, respectively. The steps to decode `message` are as follows:
*
* 1. Use the **first** appearance of all 26 lowercase English letters in `key` as the **order** of the substitution table.
* 2. Align the substitution table with the regular English alphabet.
* 3. Each letter in `message` is then **substituted** using the table.
* 4. Spaces `' '` are transformed to themselves.
*
* * For example, given key = " **hap** p **y** **bo** y"
(actual key would have **at least one** instance of each letter in the alphabet), we have the partial substitution table of (`'h' -> 'a'`, `'a' -> 'b'`, `'p' -> 'c'`, `'y' -> 'd'`, `'b' -> 'e'`, `'o' -> 'f'`).
*
* Return _the decoded message_.
*
* **Example 1:**
*
* 
*
* **Input:** key = "the quick brown fox jumps over the lazy dog", message = "vkbs bs t suepuv"
*
* **Output:** "this is a secret"
*
* **Explanation:** The diagram above shows the substitution table.
*
* It is obtained by taking the first appearance of each letter in " **the** **quick** **brown** **f** o **x** **j** u **mps** o **v** er the **lazy** **d** o **g** ".
*
* **Example 2:**
*
* 
*
* **Input:** key = "eljuxhpwnyrdgtqkviszcfmabo", message = "zwx hnfx lqantp mnoeius ycgk vcnjrdb"
*
* **Output:** "the five boxing wizards jump quickly"
*
* **Explanation:** The diagram above shows the substitution table.
*
* It is obtained by taking the first appearance of each letter in " **eljuxhpwnyrdgtqkviszcfmabo** ".
*
* **Constraints:**
*
* * `26 <= key.length <= 2000`
* * `key` consists of lowercase English letters and `' '`.
* * `key` contains every letter in the English alphabet (`'a'` to `'z'`) **at least once**.
* * `1 <= message.length <= 2000`
* * `message` consists of lowercase English letters and `' '`.
**/
public class Solution {
public String decodeMessage(String key, String message) {
StringBuilder sb = new StringBuilder();
Map temp = new HashMap<>();
char[] alphabet = new char[26];
int itr = 0;
for (char c = 'a'; c <= 'z'; ++c) {
alphabet[c - 'a'] = c;
}
for (int i = 0; i < key.length(); i++) {
if (!temp.containsKey(key.charAt(i)) && key.charAt(i) != ' ') {
temp.put(key.charAt(i), alphabet[itr++]);
}
}
for (int j = 0; j < message.length(); j++) {
if (message.charAt(j) == ' ') {
sb.append(' ');
} else {
char result = temp.get(message.charAt(j));
sb.append(result);
}
}
return sb.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy