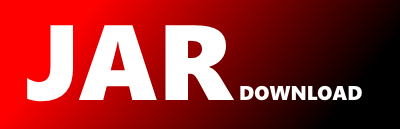
g2301_2400.s2389_longest_subsequence_with_limited_sum.Solution Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of leetcode-in-java21 Show documentation
Show all versions of leetcode-in-java21 Show documentation
Java-based LeetCode algorithm problem solutions, regularly updated
package g2301_2400.s2389_longest_subsequence_with_limited_sum;
// #Easy #Array #Sorting #Greedy #Binary_Search #Prefix_Sum
// #2022_09_02_Time_4_ms_(99.97%)_Space_42.7_MB_(95.35%)
import java.util.Arrays;
/**
* 2389 - Longest Subsequence With Limited Sum\.
*
* Easy
*
* You are given an integer array `nums` of length `n`, and an integer array `queries` of length `m`.
*
* Return _an array_ `answer` _of length_ `m` _where_ `answer[i]` _is the **maximum** size of a **subsequence** that you can take from_ `nums` _such that the **sum** of its elements is less than or equal to_ `queries[i]`.
*
* A **subsequence** is an array that can be derived from another array by deleting some or no elements without changing the order of the remaining elements.
*
* **Example 1:**
*
* **Input:** nums = [4,5,2,1], queries = [3,10,21]
*
* **Output:** [2,3,4]
*
* **Explanation:** We answer the queries as follows:
*
* - The subsequence [2,1] has a sum less than or equal to 3. It can be proven that 2 is the maximum size of such a subsequence, so answer[0] = 2.
*
* - The subsequence [4,5,1] has a sum less than or equal to 10. It can be proven that 3 is the maximum size of such a subsequence, so answer[1] = 3.
*
* - The subsequence [4,5,2,1] has a sum less than or equal to 21. It can be proven that 4 is the maximum size of such a subsequence, so answer[2] = 4.
*
* **Example 2:**
*
* **Input:** nums = [2,3,4,5], queries = [1]
*
* **Output:** [0]
*
* **Explanation:** The empty subsequence is the only subsequence that has a sum less than or equal to 1, so answer[0] = 0.
*
* **Constraints:**
*
* * `n == nums.length`
* * `m == queries.length`
* * `1 <= n, m <= 1000`
* * 1 <= nums[i], queries[i] <= 106
**/
public class Solution {
public int[] answerQueries(int[] nums, int[] queries) {
// we can sort the nums because the order of the subsequence does not matter
Arrays.sort(nums);
for (int i = 1; i < nums.length; i++) {
nums[i] = nums[i] + nums[i - 1];
}
for (int i = 0; i < queries.length; i++) {
int j = Arrays.binarySearch(nums, queries[i]);
if (j < 0) {
j = -j - 2;
}
queries[i] = j + 1;
}
return queries;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy