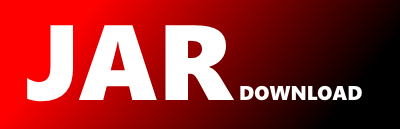
g2301_2400.s2395_find_subarrays_with_equal_sum.Solution Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of leetcode-in-java21 Show documentation
Show all versions of leetcode-in-java21 Show documentation
Java-based LeetCode algorithm problem solutions, regularly updated
package g2301_2400.s2395_find_subarrays_with_equal_sum;
// #Easy #Array #Hash_Table #2022_09_14_Time_0_ms_(100.00%)_Space_40.3_MB_(93.74%)
import java.util.HashSet;
import java.util.Set;
/**
* 2395 - Find Subarrays With Equal Sum\.
*
* Easy
*
* Given a **0-indexed** integer array `nums`, determine whether there exist **two** subarrays of length `2` with **equal** sum. Note that the two subarrays must begin at **different** indices.
*
* Return `true` _if these subarrays exist, and_ `false` _otherwise._
*
* A **subarray** is a contiguous non-empty sequence of elements within an array.
*
* **Example 1:**
*
* **Input:** nums = [4,2,4]
*
* **Output:** true
*
* **Explanation:** The subarrays with elements [4,2] and [2,4] have the same sum of 6.
*
* **Example 2:**
*
* **Input:** nums = [1,2,3,4,5]
*
* **Output:** false
*
* **Explanation:** No two subarrays of size 2 have the same sum.
*
* **Example 3:**
*
* **Input:** nums = [0,0,0]
*
* **Output:** true
*
* **Explanation:** The subarrays [nums[0],nums[1]] and [nums[1],nums[2]] have the same sum of 0.
*
* Note that even though the subarrays have the same content, the two subarrays are considered different because they are in different positions in the original array.
*
* **Constraints:**
*
* * `2 <= nums.length <= 1000`
* * -109 <= nums[i] <= 109
**/
public class Solution {
public boolean findSubarrays(int[] nums) {
Set set = new HashSet<>();
for (int i = 1; i < nums.length; ++i) {
if (!set.add(nums[i] + nums[i - 1])) {
return true;
}
}
return false;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy