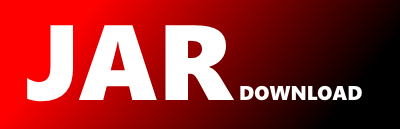
g2401_2500.s2429_minimize_xor.Solution Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of leetcode-in-java21 Show documentation
Show all versions of leetcode-in-java21 Show documentation
Java-based LeetCode algorithm problem solutions, regularly updated
package g2401_2500.s2429_minimize_xor;
// #Medium #Greedy #Bit_Manipulation #2022_12_07_Time_0_ms_(100.00%)_Space_39.6_MB_(87.47%)
/**
* 2429 - Minimize XOR\.
*
* Medium
*
* Given two positive integers `num1` and `num2`, find the positive integer `x` such that:
*
* * `x` has the same number of set bits as `num2`, and
* * The value `x XOR num1` is **minimal**.
*
* Note that `XOR` is the bitwise XOR operation.
*
* Return _the integer_ `x`. The test cases are generated such that `x` is **uniquely determined**.
*
* The number of **set bits** of an integer is the number of `1`'s in its binary representation.
*
* **Example 1:**
*
* **Input:** num1 = 3, num2 = 5
*
* **Output:** 3
*
* **Explanation:** The binary representations of num1 and num2 are 0011 and 0101, respectively. The integer **3** has the same number of set bits as num2, and the value `3 XOR 3 = 0` is minimal.
*
* **Example 2:**
*
* **Input:** num1 = 1, num2 = 12
*
* **Output:** 3
*
* **Explanation:** The binary representations of num1 and num2 are 0001 and 1100, respectively. The integer **3** has the same number of set bits as num2, and the value `3 XOR 1 = 2` is minimal.
*
* **Constraints:**
*
* * 1 <= num1, num2 <= 109
**/
public class Solution {
public int minimizeXor(int num1, int num2) {
int bits = Integer.bitCount(num2);
int result = 0;
for (int i = 30; i >= 0 && bits > 0; --i) {
if ((1 << i & num1) != 0) {
--bits;
result = result ^ (1 << i);
}
}
for (int i = 0; i <= 30 && bits > 0; ++i) {
if ((1 << i & num1) == 0) {
--bits;
result = result ^ (1 << i);
}
}
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy