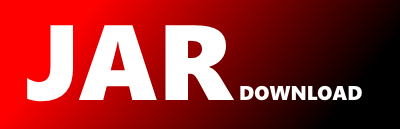
g2401_2500.s2490_circular_sentence.Solution Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of leetcode-in-java21 Show documentation
Show all versions of leetcode-in-java21 Show documentation
Java-based LeetCode algorithm problem solutions, regularly updated
package g2401_2500.s2490_circular_sentence;
// #Easy #String #2023_01_27_Time_1_ms_(99.85%)_Space_42.6_MB_(55.63%)
/**
* 2490 - Circular Sentence\.
*
* Easy
*
* A **sentence** is a list of words that are separated by a **single** space with no leading or trailing spaces.
*
* * For example, `"Hello World"`, `"HELLO"`, `"hello world hello world"` are all sentences.
*
* Words consist of **only** uppercase and lowercase English letters. Uppercase and lowercase English letters are considered different.
*
* A sentence is **circular** if:
*
* * The last character of a word is equal to the first character of the next word.
* * The last character of the last word is equal to the first character of the first word.
*
* For example, `"leetcode exercises sound delightful"`, `"eetcode"`, `"leetcode eats soul"` are all circular sentences. However, `"Leetcode is cool"`, `"happy Leetcode"`, `"Leetcode"` and `"I like Leetcode"` are **not** circular sentences.
*
* Given a string `sentence`, return `true` _if it is circular_. Otherwise, return `false`.
*
* **Example 1:**
*
* **Input:** sentence = "leetcode exercises sound delightful"
*
* **Output:** true
*
* **Explanation:** The words in sentence are ["leetcode", "exercises", "sound", "delightful"].
* - leetcode's last character is equal to exercises's first character.
* - exercises's last character is equal to sound's first character.
* - sound's last character is equal to delightful's first character.
* - delightful's last character is equal to leetcode's first character.
*
* The sentence is circular.
*
* **Example 2:**
*
* **Input:** sentence = "eetcode"
*
* **Output:** true
*
* **Explanation:** The words in sentence are ["eetcode"].
* - eetcode's last character is equal to eetcode's first character. The sentence is circular.
*
* **Example 3:**
*
* **Input:** sentence = "Leetcode is cool"
*
* **Output:** false
*
* **Explanation:** The words in sentence are ["Leetcode", "is", "cool"]. - Leetcode's last character is **not** equal to is's first character. The sentence is **not** circular.
*
* **Constraints:**
*
* * `1 <= sentence.length <= 500`
* * `sentence` consist of only lowercase and uppercase English letters and spaces.
* * The words in `sentence` are separated by a single space.
* * There are no leading or trailing spaces.
**/
public class Solution {
public boolean isCircularSentence(String sentence) {
char[] letters = sentence.toCharArray();
int len = letters.length;
for (int i = 0; i < len - 1; ++i) {
if (letters[i] == ' ' && letters[i - 1] != letters[i + 1]) {
return false;
}
}
return letters[0] == letters[len - 1];
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy