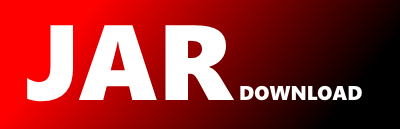
g2501_2600.s2594_minimum_time_to_repair_cars.Solution Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of leetcode-in-java21 Show documentation
Show all versions of leetcode-in-java21 Show documentation
Java-based LeetCode algorithm problem solutions, regularly updated
package g2501_2600.s2594_minimum_time_to_repair_cars;
// #Medium #Array #Binary_Search #2023_08_23_Time_15_ms_(97.28%)_Space_53.8_MB_(85.07%)
/**
* 2594 - Minimum Time to Repair Cars\.
*
* Medium
*
* You are given an integer array `ranks` representing the **ranks** of some mechanics. ranksi is the rank of the ith mechanic. A mechanic with a rank `r` can repair n cars in r * n2
minutes.
*
* You are also given an integer `cars` representing the total number of cars waiting in the garage to be repaired.
*
* Return _the **minimum** time taken to repair all the cars._
*
* **Note:** All the mechanics can repair the cars simultaneously.
*
* **Example 1:**
*
* **Input:** ranks = [4,2,3,1], cars = 10
*
* **Output:** 16
*
* **Explanation:**
*
* - The first mechanic will repair two cars. The time required is 4 \* 2 \* 2 = 16 minutes.
*
* - The second mechanic will repair two cars. The time required is 2 \* 2 \* 2 = 8 minutes.
*
* - The third mechanic will repair two cars. The time required is 3 \* 2 \* 2 = 12 minutes.
*
* - The fourth mechanic will repair four cars. The time required is 1 \* 4 \* 4 = 16 minutes.
*
* It can be proved that the cars cannot be repaired in less than 16 minutes.
*
* **Example 2:**
*
* **Input:** ranks = [5,1,8], cars = 6
*
* **Output:** 16
*
* **Explanation:**
*
* - The first mechanic will repair one car. The time required is 5 \* 1 \* 1 = 5 minutes.
*
* - The second mechanic will repair four cars. The time required is 1 \* 4 \* 4 = 16 minutes.
*
* - The third mechanic will repair one car. The time required is 8 \* 1 \* 1 = 8 minutes.
*
* It can be proved that the cars cannot be repaired in less than 16 minutes.
*
* **Constraints:**
*
* * 1 <= ranks.length <= 105
* * `1 <= ranks[i] <= 100`
* * 1 <= cars <= 106
**/
public class Solution {
public long repairCars(int[] ranks, int cars) {
long low = 0;
long high = 100000000000000L;
long pivot;
while (low <= high) {
pivot = low + (high - low) / 2;
if (canRepairAllCars(ranks, cars, pivot)) {
high = pivot - 1;
} else {
low = pivot + 1;
}
}
return low;
}
private boolean canRepairAllCars(int[] ranks, int cars, long totalMinutes) {
int repairedCars = 0;
for (int i = 0; i < ranks.length && repairedCars < cars; i++) {
repairedCars += (int) Math.sqrt((double) totalMinutes / ranks[i]);
}
return repairedCars >= cars;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy