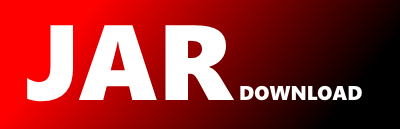
g2601_2700.s2680_maximum_or.Solution Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of leetcode-in-java21 Show documentation
Show all versions of leetcode-in-java21 Show documentation
Java-based LeetCode algorithm problem solutions, regularly updated
package g2601_2700.s2680_maximum_or;
// #Medium #Array #Greedy #Bit_Manipulation #Prefix_Sum
// #2023_09_11_Time_2_ms_(100.00%)_Space_55.2_MB_(56.19%)
/**
* 2680 - Maximum OR\.
*
* Medium
*
* You are given a **0-indexed** integer array `nums` of length `n` and an integer `k`. In an operation, you can choose an element and multiply it by `2`.
*
* Return _the maximum possible value of_ `nums[0] | nums[1] | ... | nums[n - 1]` _that can be obtained after applying the operation on nums at most_ `k` _times_.
*
* Note that `a | b` denotes the **bitwise or** between two integers `a` and `b`.
*
* **Example 1:**
*
* **Input:** nums = [12,9], k = 1
*
* **Output:** 30
*
* **Explanation:** If we apply the operation to index 1, our new array nums will be equal to [12,18]. Thus, we return the bitwise or of 12 and 18, which is 30.
*
* **Example 2:**
*
* **Input:** nums = [8,1,2], k = 2
*
* **Output:** 35
*
* **Explanation:** If we apply the operation twice on index 0, we yield a new array of [32,1,2]. Thus, we return 32|1|2 = 35.
*
* **Constraints:**
*
* * 1 <= nums.length <= 105
* * 1 <= nums[i] <= 109
* * `1 <= k <= 15`
**/
public class Solution {
public long maximumOr(int[] nums, int k) {
int[] suffix = new int[nums.length];
for (int i = nums.length - 2; i >= 0; i--) {
suffix[i] = suffix[i + 1] | nums[i + 1];
}
long prefix = 0L;
long max = 0L;
for (int i = 0; i <= nums.length - 1; i++) {
long num = nums[i];
max = Math.max(max, prefix | (num << k) | suffix[i]);
prefix |= num;
}
return max;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy